CSC/ECE 517 Fall 2007/wiki3 3 aa
Topic
Take the Interface Segregation principle and catalog the information on it available on the Web. We didn't cover it in class, but you can look it up on the Web or in the ACM DL. Find good descriptions and good, concise, understandable examples. Tell which you consider the best to present to a class.
Interface Segregation Principle (ISP)
The Interface Segregation Principle states that "Clients should not be forced to depend upon interfaces that they do not use".
ISP in OO Design
When clients are forced to depend upon interfaces that they don’t use, then those clients are subject to changes to those interfaces. This results in an inadvertent coupling between all the clients. Said another way, when a client depends upon a class that contains interfaces that the client does not use, but that other clients do use, then that client will be affected by the changes that those other clients force upon the class. This relationship is illustrated below, where Fig.1 indicates OO Design without ISP and Fig.2 indicates OO Design with ISP. We would like to avoid such couplings where possible, and so we want to separate the interfaces where possible. ISP is about high cohesion in the interface level. It is often referred to as poor man's Single Responsibility Principle (SRP) because SRP deals with cohesion at the class level.

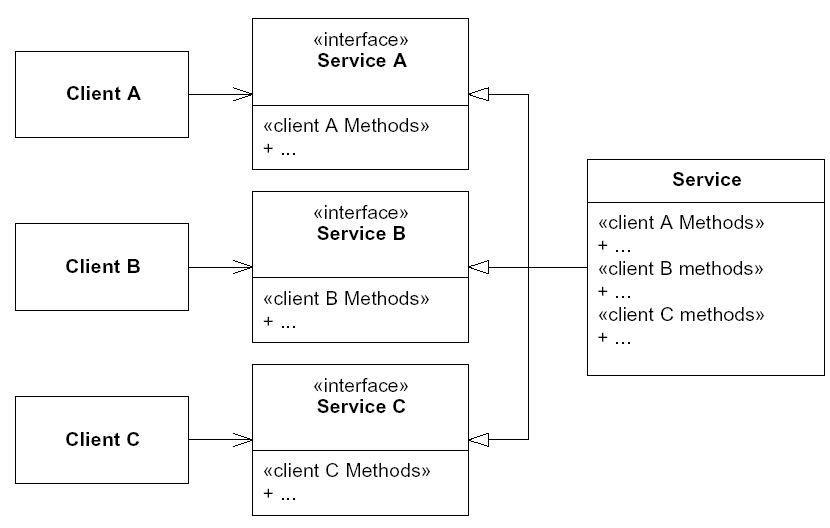
Without ISP, the OO Design may contain fat interfaces. "Fat" interfaces arise from classes whose member methods can be broken into two or more groups. Each group of methods in turn serves a different set of clients. The Interface Segregation Principle suggests that client should not know about the method groups as a single class. Instead, clients should know about abstract base classes, one for each method group, which have cohesive interfaces. Fat interfaces lead to inadvertent couplings between clients that ought otherwise to be isolated. By making use of the ADAPTER pattern, either through delegation (object form) or multiple inheritance (class form), fat interfaces can be segregated into abstract base classes that break the unwanted coupling between clients.
The ISP acknowledges that there are objects that require non-cohesive interfaces; however it suggests that clients should not know about them as a single class. Instead, clients should know about abstract base classes that have cohesive interfaces. Some languages refer to these abstract base classes as “interfaces”, “protocols” or “signatures”.
Examples of ISP
Example 1
The following example is a very easy to understand example of how ISP can be employed in a real world system design.
Consider a cell phone had interfaces of a phone and a MP3 player. ISP advises to keep these two interfaces completely independent of each other. This way the phone can be treated purely as a phone or as a MP3 player. Programmatically,use only the interface of a MP3 player in an implemented method called playSong. In future even if the interface for the phone is modified, the method playSong would remain the same.
This example can be used to give a general overview of ISP, without going into much detail of how ISP is used in making complex OO design decision.
Example 2
The following example is another good example that shows how ISP is used to deal with fat interfaces. In the illustrations below we have made use of UML (Unified Modeling Language) to bring out the design decisions that we have made.
Consider a security system. In this system there are Door objects that can be locked and unlocked, and which know whether they are open or closed.
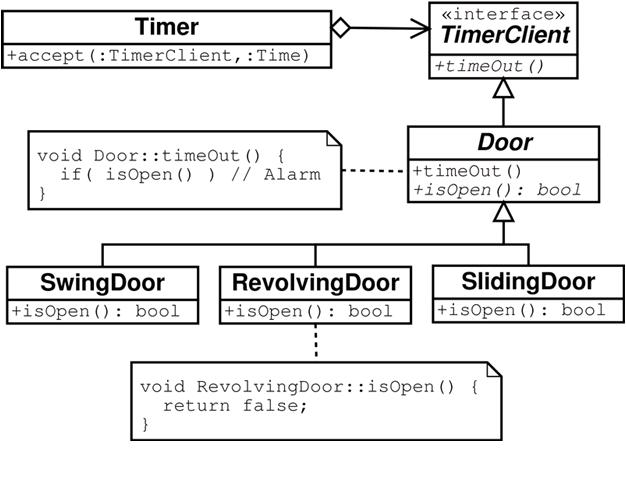

Example 3

Example 4
Consider a slightly more significant example. The traditional Automated Teller Machine (ATM) problem. The user interface of an ATM machine needs to be very flexible. The output may need to be translated into many different language. It may need to be presented on a screen, or on a braille tablet, or spoken out a speech synthesizer. Clearly this can be achieved by creating an abstract base class that has pure virtual functions for all the different messages that need to be presented by the interface. Fig.6 Represents a general UI design for the ATM.
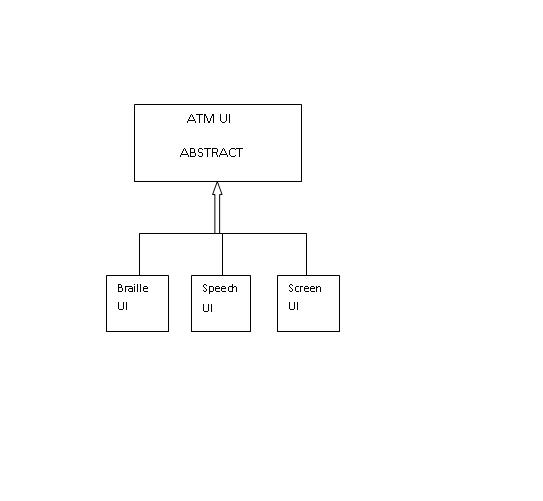
Also consider that different transactions that the ATM can perform is encapsulated as a derivative of the class Transaction. Thus we might have classes such as DepositTransaction, WithdrawlTransaction, TransferTransaction, etc. Each of these objects issues message
to the UI. For example, the DepositTransaction object calls the RequestDepositAmount member function of the UI class. Whereas the transferTransaction object calls the RequestTransferAmount member function of UI. This corresponds to the diagram in Figure 7.
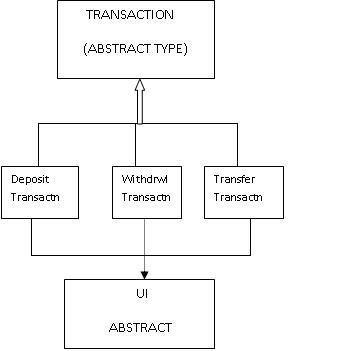
Notice that this is precicely the situation that the ISP tells us to avoid. Each of the transactions is using a portion of the UI that no other object uses. This creates the possibility that changes to one of the derivatives of Transaction will force coresponding change to the UI, thereby affecting all the other derivatives of Transaction, and every other class that depends upon the UI interface.
This unfortunate coupling can be avoided by segregating the UI interface into induvidual abstract base classes such as DepositUI, withdrawUI and TransferUI. These abstract base classes can then be multiply inherited into the final UI abstract class. Fig.8 show this model.
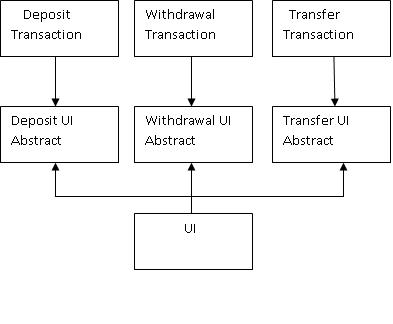
Catalog
http://www.objectmentor.com/resources/articles/isp.pdf http://doodleproject.sourceforge.net/articles/2001/interfaceSegregationPrinciple.html http://ifacethoughts.net/2006/03/28/interface-segregation-principle/ http://www.everything2.com/index.pl?node_id=1259576 http://www.cmcrossroads.com/articles/agile-cm-environments/principles-of-agile-version-control:-from-ood-to-tbd.html http://jayflowers.com/WordPress/?p=91 http://www.cet.sunderland.ac.uk/~cs0her/COM379%20Lectures/Lecture3.ppt http://www.parlezuml.com/metrics/OO%20Design%20Principles%20&%20Metrics.pdf