CSC/ECE 517 Spring 2017/final T1701
Introduction
What is Tago?
Founded in 2014,Tago is startup based out of Centennial Campus. They have created a service that allows users to craft dashboards that are used by Internet of Things (IoT) devices for a variety of purposes. These include storing/visualizing data, running triggers that send emails, SMS messages, or data to other devices/http endpoints, and initiating custom analysis scripts to manipulate and analyze data. Along with the dashboard, Tago has developed an SDK that developers can utilize when programming on the IoT devices in order to make these various requests.
What are we doing?
Currently, Tago has a node.js-SDK, but they have begun work on a Python SDK. We have decided to assist in order to speed up the the development and delivery of their Python SDK. We have broken down the project into two teams, T1701 and T1702, and split the work by assigning different subsets of functionality. It is worth noting that we are building a Python interface for interacting with Tago’s RESTful API, not a web-based UI or a web-app. The main goal of the project is to port the current SDK written in NodeJS to one that supports Python.

Why are we doing it?
Tago currently has customers with needs for a Python SDK including Texas Instruments and Tesla. The sooner the SDK is developed, the sooner these companies can utilize them. Python has a number of advantages over NodeJS, particularly around the communities. The Python community has implemented a vast number of scientific and other niche libraries that could be useful in IoT sensors and measurement equipment.
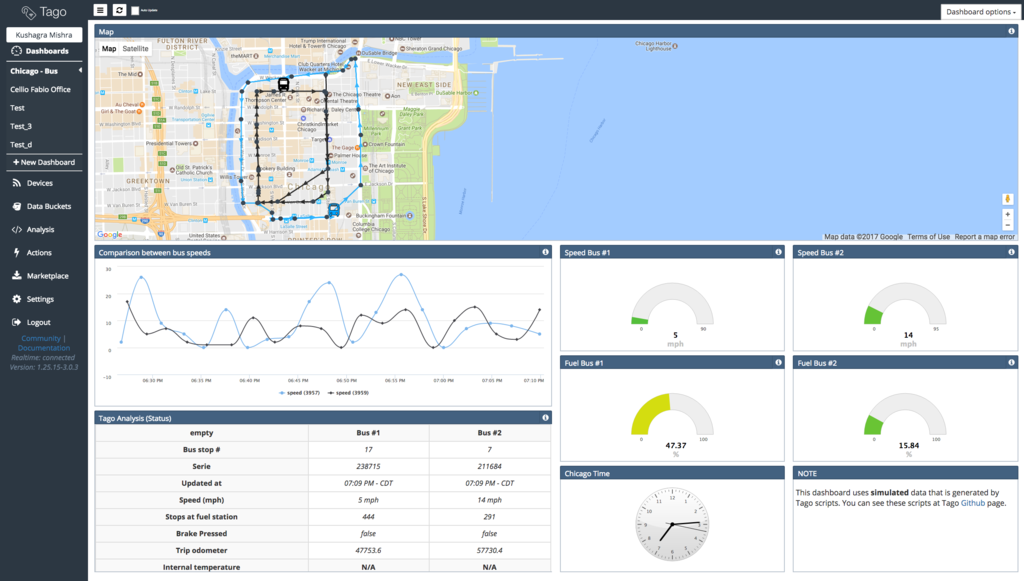
Design
The SDK is broken into several modules, each of which represents a class. The class diagram shown below represents each of these classes and their methods. This project deals with the services and the extra modules.
The SDK provides services module to enhance support for the application. These services can be triggered when the customer application wants to send an alert or update the customer about the status of the IoT system.
The extra module provides some 3rd party functionalities which the customer can use in their application. Like the geolocation feature can be used along with GPS sensor to pin point a location on the map.
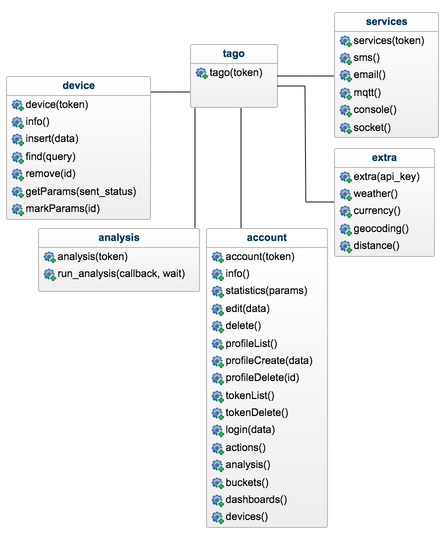
Implementation
As can be seen in the design, there are plenty of modules in tago-sdk. The services and extra API modules are being implemented in Python (currently implemented using NodeJS) as a part of this project. These modules will interact with Tago servers and third-party vendors to serve HTTP requests to clients.
In the following, a Promise is simply an object used for asynchronous computations, which represents the resulting status of the operation (success or failure), with each scenario having an appropriate callback function.
Services
As a part of services, Tago should provide some functions that can greatly help your application. When setting up a service, an analysis-token (token is analogous to API key) needs to be passed. For convenience, the context returns a property token that you can use to setup a service object.
sms
The sms class should provide support to send a sms directly to tago admins or customers from analysis. SMS costs incurred depend on the country of operation.
Method
- send
- This method is used to send SMS.
- Arguments
- Phone number. (With the country code if not in the US)
- Message to be sent
The email class should provide support to send a email with analysis details or any other info.
Method
- send
- This method is used to send email.
- Arguments
- Email address
- Subject of the email
- Message to be sent
- E-mail address for the receiver to reply to
mqtt
The mqtt class should provide a simple way to publish some data to the Tago broker.
Method
- publish
- This method is used by the client to publish to the Tago broker. Check out MQTT and MQTT in Tago for more information on how it works.
- Arguments
- message
- bucket
socket
The socket class should provide support to send some data to a bucket via an HTTP POST call.
Method
- send
- This method is used to send some JSON data to the bucket.
- Arguments
- bucket_id
- data_entry
console
The console class should provide support to send some logged data to the Tago server.
Method
- send
- This method is used to send some message with a time-stamp to the server.
- Arguments
- message
- timestamp
Third Party APIs (Extra)
Tago supports third-party API’s, like Google Maps and Weather service. All third-party services are free to use, with a free token(API Key) that can obtained from the third party website.
geocoding
This class is used to get a geolocation (latitude/longitude) for a valid address, or vice versa. To make use of this functionality, a google geocoding API key needs to be provided while creating a new object. Geocoding API provider documentation.
Methods
- getGeolocation
- Convert the address to a valid geolocation, if it exists
- Arguments
- address(string) A valid address
- Returns
- (Promise)
- getAddress
- Convert a valid geolocation to an address, if it exists.
- Arguments
- geolocation(string) A valid geolocation
- Returns
- (Promise)
currency
This class checks several currencies in real-time, and the historical exchange rates for over 168 countries. Currency API provider
Methods
- convert
- Returns the current exchange rate of one currency to another one
- Arguments
- from(string) convert from. See supported currencies for more information
- Returns
- (Promise)
distance
This class helps to calculate the distance between two points. Google Distance API
Methods
- measure
- Measure is a service that provides the travel distance and time for a matrix of origins and destinations.
- Arguments
- origins(array) An array of origins, can be string location or geojson.
- destinations(array) An array of destinations, can be string location or geojson.
- language(string) Set a language. Default is ‘EN’. See language support for more information. (optional)
- mode(string) For the calculation of distances, you may specify the transportation mode to use. By default, distances are calculated for driving mode. See the travel modes supported for more information
- Returns
- (Promise)
weather
Whenever you need to get weather conditions around the world, use weather service APIs. This class defines helper methods to achieve this and talk to tago server. Wunderground API
Methods
- current
- Get the current weather conditions.
- Arguments
- query(string) It can be address, zipcode or geojson.
- full(boolean) Set to get response with full description. Default is false. (optional)
- language(string) Set the language. Default is ‘EN’. See language support for more information. (optional)
- Returns
- (Promise)
- forecast
- Returns a summary of the weather forecast for the next 10 days. This includes high and low temperatures, a string text forecast and other conditions.
- Arguments
- query(string) It can be address, zipcode or geojson.
- full(boolean) Set to get the response with full description. Default is false. (optional)
- language(string) Set the language. Default is ‘EN’. See `language support language support for more information. (optional)
- Returns
- (Promise)
- history
- Returns a summary of the weather conditions for the last 10 days. This includes high and low temperatures, a string text and other weather conditions.
- Arguments
- date(string) a past date.
- query(string) It can be address, zipcode or geojson.
- full(boolean) Set to get response with full description. Default is false. (optional)
- language(string) Set the language. Default is ‘EN’. See language support for more information. (optional).
- Returns
- (Promise)
- alert
- Returns the short name description, expiration time and a long text description of a severe alert, if one has been issued for the searched location.
- Arguments
- query(string) It can be address, zipcode or geojson.
- full(boolean) Set to get response with full description. Default is false. (optional)
- language(string) Set a language. Default is ‘EN’. See language support for more information. (optional).
- Returns
- (Promise)
Test Plan
Test Type | Unit Test |
Testing Tool | pytest and requests-mock
This requests-mock module stubs out HTTP request part of the testing code. |
Services Module | ![]() |
Description |
Each of the classes and function in the Services module will be tested in the following manner (eg. function testing SMS.send):
|
Extra Module | ![]() |
Description |
Each of the classes and function in the Extra module will be tested in the following manner (eg. function testing Weather.forecast):
|
Test Type | Integration Test |
Testing Tool | pytest |
Extra Module | ![]() |
Description |
Each of the classes and function in the Extra module will be tested in the following manner (eg. function testing Weather.forecast):
|
Testing Tool | Manual Testing |
Service Module | The manual testing will be done by providing example scripts.
For sms method, the tester can add his/her number and run the script and test whether sms is being sent or not. Similarly, for the email method the tester can add his/her email address and test whether email is sent or not after running the script. |
Extra Module | Example scripts will be provided which will use the third party API calls using the SDK and print the response on console. |
Running Tests
In order to run the unit and integration tests on your system, follow these steps:
- Clone the repo:
- Then set environment variable
- export TAGO_TOKEN_DEVICE=6ea148d0-e75c-11e6-a091-5756b91044c4
- From the top folder run
- python setup.py test