CSC/ECE 517 Spring 2013/ch1a 1e pi
Inheritance
In object-oriented programming (OOP), inheritance is a way to reuse code of existing objects, or to establish a subtype from an existing object, or both
Means of achieving inheritance
Classical Inheritance
Objects are defined by classes, classes can inherit attributes and behavior from pre-existing classes called base classes, superclasses, or parent classes. The resulting classes are known as derived classes, subclasses, or child classes. The relationships of classes through inheritance gives rise to a hierarchy.
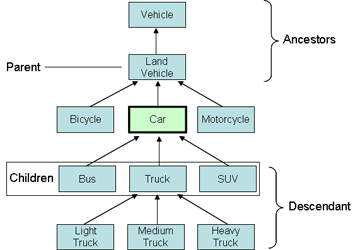
Prototype Based Inheritance(1)
A feature of object-oriented programming in which classes are not present and inheritance is performed via a process of cloning(3) existing objects that serve as prototypes . Delegation(2) is the language feature that supports prototype-based programming.
A simple example of Prototype Based Inheritance could be depicted as
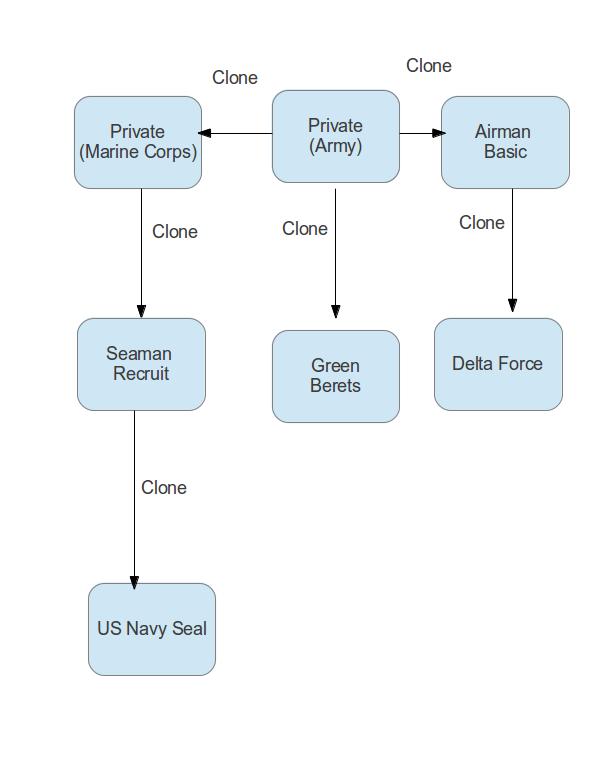
.
(For the sake of an example, do not assume any of the military categories mentioned to be a class. They are all objects. For example we will not create an object for Green Berets. Rather Green Berets is itself an object. This has been done to avoid the use of common names for a soldier and then assigning his type to be a private or a green beret or a navy seal, etc)
This example shows soldiers belonging to different divisions as different objects. This sort of a relation can be better be represented as a prototype based inheritance than as a class-based one. If the relation used was the classical approach, then a separate class "soldier" would have to be defined as the base class from which the rest of the classes such as delta, green berets and navy seals would have to be defined as children classes. Also these would have been the children classes from which the object itself(probably a real soldier) would have to be instantiated. There is no reason to do so in this case as, each of these, intuitively suit more as an object(real life) than as a passive conceptual class. Besides, the classical approach would bring about a hierarchy among the objects when none exists.
Classical Inheritance v/s Prototype based inheritance
Object Creation(1)
While in the case of the classical approach, objects need to be instantiated after defining the format of the class, in case of prototype based, a new object can be instantiated by simply creating a copy of/cloning an existing object. As in
Classical inheritance example class Vehicle { //properties } class Car inherits Land Vehicle { } class trucks inherits inherits Car { } class medium Truck inherits Truck { //properties }
Prototype based inheritance example
class Soldier { //properties } Private = Soldier.new() GreenBeret.prototype = Private GreenBeret.weapon=("H&K") GreenBeret.training=("x months")
Using Preexisting modules(5)
Prototypes are more concrete than classes because they are examples of objects rather than descriptions of format and initialization. These examples may help users to reuse modules by making them easier to understand. A prototype-based system allows the user to examine a typical representative rather than requiring him to make sense out of its description
Support for one-of-a-kind objects(5)
Ruby provides a framework that can easily include one-of-a-kind objects with their own behavior. Since each object has named slots, and slots can hold state or behavior, any object can have unique slots or behavior. Class-based systems are designed for situations where there are many objects with the same behavior. There is no linguistic support for an object to possess its own unique behavior, and it is awkward to create a class that is guaranteed to have only one instance. Ruby suffers from neither of these disadvantages. Any object can be customized with its own behavior. A unique object can hold the unique behavior, and a separate "instance" is not needed.
In the above soldier example, navy seals may be trained in marine combat techniques which makes the navy seal object have its own unique behavior. Similarly a private in the army may be trained to handle tanks, (if he were to be in the cavalry division) which makes it unique for the private(army) object. In a class based approach,if private(marine corps) were to be inherited from private(army) and navy seals inherit from private(marine corps) then it would mean that the navy seal would be trained in tanks too, which is not the case here. However in prototype based inheritance training in tanks can remain unique to the marine corps if it is do desired.
Elimination of meta-regress(4)
No object in a class-based system can be self-sufficient; another object (its class) is needed to express its structure and behavior. On the other hand, in prototype-based systems an object can include its own behavior; no other object is needed to breathe life into it. Prototypes eliminate meta-regress.
For example in case of the soldier, a Delta force unit is its own object. It does not require a an airman basic object to define any of its properties even though it inherits from the same. However in case of the vehicle example, a medium truck cannot be an object of its own completely. It would need some of its properties to be defined by its superclass 'vehicle'. Consider the move(direction, speed) function defined for the Vehicle class. In most of the cases the medium truck would inherit this functionality completely. It is not likely to have its own move() function.
Transfer of state information on extending the functionality of an object(5)
Using prototype-based programming, we are able to treat any object as a prototype and therefore we can extend the object without having to create a new object. In class-based programming, we are committed to the functionality provided by the object at instantiation. If we later need to extend the functionality of the class-based object, we must instantiate a new class-based object that has the desired functionality and transfer state between the two objects.
Consider the transfer of a private from marine corps to green berets. In the prototype-based solution, we simply extend the marine corps object to have the functionality of a Green Beret. In a class-based solution, we must instantiate a new Green Beret object and transfer state from the existing marine corp object to the new Green Beret object.
Instances can become templates for other instances in case of Protoype-based Inheritance, not so in class-based(5)
if you want to clone an object it is easy, just use the existing object as the prototype for the new object. No need to write lots of complex cloning-logic for different classes.
For example, suppose a new program is being introduced to train a junior delta soldier in jungle warfare (say deltaJ), then we simply create a new deltaJ object by cloning an existing delta soldier(using it as a template) in case of Prototype-based inheritance. However in a class based approach, we would have to first create a new subclass deltaJ, instantiate an object of the new class and then write the code to clone the existing delta soldier.
Prototype based inheritance is more suited for a "functional" style of programming(5)
In case of prototype-inheritance we write lots of functions that analyse objects and act appropriately, rather than embedded logic in methods attached to specific classes.
Consider the case where a private in the army may be trained with handling an anti-tank weapon or may be trained for handling artillery. This would be impossible in the class based approach since a private would be a class and only those properties which are generic can be embedded in the private's class logic. However in case of the prototype approach, we already know the object so we define the appropriate function for the object.
Prototype based inheritance requires dynamic typing whereas the classical based approach does not(4)
Since objects are being inherited rather than classes, it is necessary that the language support dynamic typing, otherwise there is no way to determine whether the object that is inheriting from another is eligible to possess certain properties.This in turn means increased overhead, as each time an object's functionality is executed, it must be ensured that the object is of a type that has the required functionality.
In the above example, a green beret has been cloned from a private(army). However, if at runtime the green beret is cloned from an airman basic, then all its properties need to reflect those of the new parent. If the language was static then this would simply throw a compiler error (unless it supported runtime polymorhism).
Refactorings don't work the same way(5)
In a prototype based system we are essentially "building" our inheritance heirarchy with code. IDEs /refactoring tools can't really help us since they can't guess our approach.
Consider the vehicle example. Suppose the Car class had a field "type_of_fuel_used". This would not be appropriate as every instance of each of its subclass would use a different kind of fuel. Hence it would be better if the field, its setters and getters were moved to the subclass. This would be a push down refactoring which can be achieved by any IDE. Once the push down has occured any object instantiated from one of the 3 classes Bus, Truck or SUV would have its own member variable and the corresponding getter and setter. Now consider the soldier example. Suppose some of the objects - the private, airman, green beret and delta are part of the same division sent out on the same mission. Thought the mission is the same, each of them would have a different objective. Thus if objective was a field member of the private object, it would have to be pushed down to all who have cloned from it. This however the IDE would not be able to do as cloning occurs at runtime and there is no way to keep track of all the cloned objects to which the member has to be pushed.
Static typing assurances(5)
The classical approach offers static typing wherein the type of an object is determined at compile time. Since all languages that allow prototype based inheritance are dynamically typed, it implies more test cases need to be tested to ensure correct behavior for the latter approach.
Which is the better approach(5)
The classical based method is more traditional and purist compared to the prototype based approach. People used to a conventional OOP style may easily get confused. "What do you mean there's only one class called "Thing"?!?" - "How do I extend this final Thing class!?!" - "You are violating OOP principles!!!" - "It's wrong to have all these static functions that act on any kind of object!?!?" It is really the type of software, objects that are interacting with each other and functionality that determine which approach needs to be used.
For instance, if the above soldier example were about an army rather than individual or special divisions in the army then the classical approach would have been more appropriate, as there is already a natural existing hierarchy within the army that can be exploited. It would seem vague if different ranks such as lieutenant, corporal, major, general, etc were to be created as objects rather than classes. Also since each of these ranks can have multiple instances of real world objects associated with them, performing almost exactly the same functions, it would be better to create the ranks as classes and then instantiate each soldier as an object of the respective class.
References
<references/>
(1)Prototype-based_programming (2)Delegation_(programming) (3)Cloning_(programming) (4)Work by students from previous semesters (5)advantages-of-prototype-based-oop-over-class-based-oop (6)Prototype based inheritance in javascript