CSC/ECE 517 Fall 2017/E1759
This wiki page is the description of tests performed under E1759 OSS Assignment - Test surveys and survey deployment, for Fall 2017, CSC/ECE 517.
Introduction
Expertiza
Expertiza is an open source project based on Ruby on Rails framework. Expertiza allows the instructor to create new assignments and customize new or existing assignments. It also allows the instructor to create a list of topics the students can sign up for. Students can form teams in Expertiza to work on various projects and assignments. Students can also peer review other students' submissions. Expertiza supports submission across various document types, including the URLs and wiki pages.
Setting Up The Working Environment
One of several ways to set up the environment and the one we adopted is:-
Ubuntu-Expertiza image (.OVA) [Recommended]
- This is the link for the image. (https://drive.google.com/a/ncsu.edu/file/d/0B2vDvVjH76uEUmNKVncxRUhUVVE/view?usp=sharing)
- And you can install VirtualBox (free) and import this image into VirtualBox.
Some machine may require you to enable virtualization and then run the following commands.
cd expertiza
bash ./setup.sh
bundle install
rake db:migrate
- For logging in as an instructor:-
- Username: instructor6
- Password: password
Expertiza Survey Design
Expertiza should be able to distribute surveys to the users.
The survey can be one of the following: 1. Course survey (all the participants of the course can take it). 2. Global survey (all the users in Expertiza can take it).
The survey can also be targeted (the admin can specify a group of people who will receive this survey).
The three kinds of surveys in this project are:
1. Assignment Survey questionnaire
2. Global survey questionnaire
3. Course evaluation questionnaire
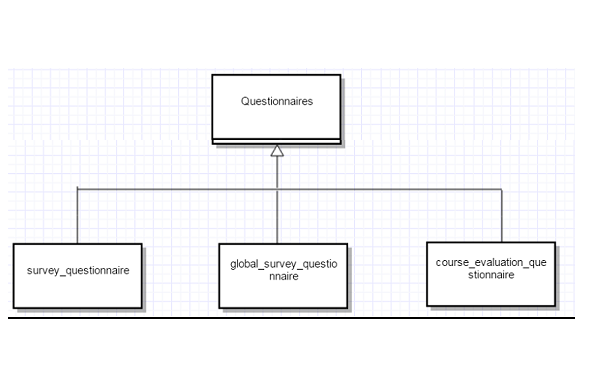
Tasks Assigned
The following tasks were performed by us as part of our project:
- Understood the flow of the survey creation and deployment function.
- Updated the RSpec file in /spec/features/ folder.
- Used fixtures to create the student record in test DB.
- Used Capybara to write functional tests for:
- Creating a new survey.
- Creating different types of questions.
- Editing different types of questions.
- Deleting different types of questions.
- Deploying survey.
- Created multiple tests to check valid and invalid cases. For example, to test valid_start_end_time? by checking that if the start time is later than the end time, the test returns false.
Files modified
- spec/features/survey_spec.rb
- spec/features/assignment_survey_spec.rb
- spec/features/global_survey_spec.rb
- spec/features/course_survey_spec.rb
- spec/features/helpers/instructor_interface_helper.rb
Test Plan (Implementation of Functional Tests)
Login as an instructor:
1. Use credentials username: instructor6, password: password.
2. Click on Manage -> Questionnaires-> Survey / Global Survey/ Course evaluation.
3. Create a new Survey/ Global Survey/ Course evaluation filling in the parameters asked for.
4. Test if the survey is created and deployed and the questions can be added, deleted and modified.
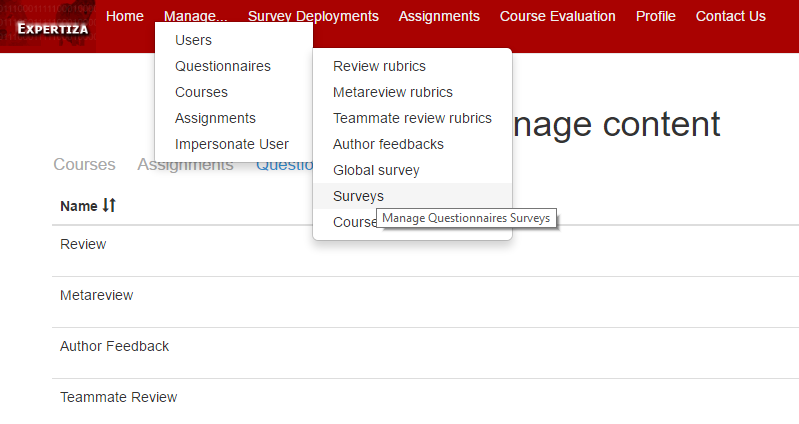
Code Snippets
The following test spec has been written to create an assignment survey.
def create_assignment_questionnaire survey_name visit '/questionnaires/new?model=Assignment+SurveyQuestionnaire&private=0' fill_in 'questionnaire_name', with: survey_name find('input[name="commit"]').click end
The following test spec has been written to deploy an assignment survey.
def deploy_assignment_survey(start_date, end_date, survey_name) login_as('instructor6') expect(page).to have_content('Manage content') create_assignment_questionnaire survey_name survey = Questionnaire.where(name: survey_name) visit '/survey_deployment/new?id=' + Assignment.where(instructor_id: User.where(name: 'instructor6').first.id).first.id.to_s + '&type=AssignmentSurveyDeployment' expect(page).to have_content('New Survey Deployment') fill_in 'survey_deployment_start_date', with: start_date fill_in 'survey_deployment_end_date', with: end_date select survey.name, from: "survey_deployment_questionnaire_id" find('input[name="commit"]').click end
The following test spec has been written to create a course survey.
def create_course_questionnaire survey_name visit '/questionnaires/new?model=Course+SurveyQuestionnaire&private=0' fill_in 'questionnaire_name', with: survey_name find('input[name="commit"]').click end
The following test spec has been written to deploy a course survey.
def deploy_course_survey(start_date, end_date, survey_name) # Here we login as an instructor to deploy a course survey. login_as('instructor6') expect(page).to have_content('Manage content') create_course_questionnaire survey_name survey = Questionnaire.where(name: survey_name) instructor = User.where(name: 'instructor6').first course = Course.where(instructor_id: instructor.id).first visit '/survey_deployment/new?id=' + course.id.to_s + '&type=CourseSurveyDeployment' expect(page).to have_content('New Survey Deployment') fill_in 'survey_deployment_start_date', with: start_date fill_in 'survey_deployment_end_date', with: end_date select survey.name, from: "survey_deployment_questionnaire_id" find('input[name="commit"]').click end
Conclusion
After completion of this project, the creation, deployment, and deletion of surveys will be fully tested.