CSC/ECE 517 Fall 2014/ch1a 26 gn
Debugging in Rails using Pry
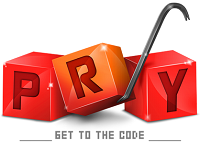
Ruby on Rails is a Web application framework for Ruby. It was first released to the public in July 2004. Within months, it was a widely used development environment. Many multinational corporations are using it to create Web applications. It is the standard Web-development framework for Ruby. Models are objects that represent the components of an application that perform information processing in the problem domain. Views are objects that display some aspect of the model. They are the output mechanism for the models. Controllers are objects that control how user actions are interpreted. They are the input mechanism for the views and models.
Since a Rails application goes beyond the model view controller, like Mailers, Routes (REST, HTTP-Verbs, Constraints), Environments and Initializers, Caching (Redis ,Memcached), Assets (CSS, SASS, Javascript, CoffeeScript, Pipelining), Bundler and dependency management, Tests (RSpec , Capybara<ref>https://github.com/jnicklas/capybara</ref>), gems, plugins and engines used in the application, one needs to know a lot of stuff to master debugging their own application<ref>http://nofail.de/2013/10/debugging-rails-applications-in-development/</ref>. Debugging is one of the things a developer has to do most and there exists debuggers for Rails that are suitable for browsers and lack the ability to allow moving around in the call-stack and inspecting objects at runtime.
Pry in literal sense means to look or inquire closely, curiously, or impertinently. Pry is a powerful alternative to the standard IRB shell for Ruby. It not only for debugging but also (and mainly) for inspecting objects. It features Syntax highlighting, a flexible plugin architecture, runtime invocation and source and documentation browsing. It enables the user to browse source code, inspect objects, eliminates all the iteration - and startup time when running red/green cycles and is especially useful when you don’t know exactly how something should function in the first place.
Pry is written in Ruby<ref>http://en.wikipedia.org/wiki/Pry_(software)</ref>. The Pry core team consists of John Mair, Conrad Irwin and Ryan Fitzgerald and many more contributors. The last stable release was version 0.9.10 and it was released on July 15, 2012.
Introduction
Pry: super-fast, painless, debugging for the (ruby) masses.
Pry aims to be more than an IRB replacement; it is an attempt to bring REPL driven programming to the Ruby language. Pry is also fairly flexible and allows significant user customization making it a good choice for implementing custom shells. The pry console gives you access to the method that raised the exception, you can use it to inspect the values of variables (no more print statements!), the source code of methods (no more flapping around with a text editor!), and even move up and down the call stack (like a real debugger!).
Because the shell opens as though the exception were just about to be raised, you don't even need to re-run your program from the beginning in order to start debugging. It's optimized for the "exceptions-happen" workflow that you need when developing code.
Features
Pry is written from scratch to provide a number of advanced features, some of these include<ref>http://pryrepl.org</ref><ref>http://andywenk.tumblr.com/post/23821244145/rails-debugging-with-pry</ref>:
- Source code browsing (including core C source with the pry-doc gem)
- Navigation around state (cd, ls and friends)
- Rubinius core source browsing
- Documentation browsing
- Live help system
- Open methods in editors (edit-method Class#method)
- Syntax highlighting
- Command shell integration (start editors, run git, and rake from within Pry)
- Gist integration
- Runtime invocation (use Pry as a developer console or debugger)
- Exotic object support (BasicObject instances, IClasses, ...)
- A powerful and flexible command system
- Ability to view and replay history
- Many convenience commands inspired by IPython, Smalltalk and other advanced REPLs
Installation
The steps you have to do are simple<ref>http://www.sitepoint.com/pry-friends-rails</ref>:
$ gem install pry $ pry
You could install pry on Rails by adding the pry-rails gem to your gem file:
group :development do gem 'pry' ... end
Once you have got that settled, change back into the sample_app directory and install all the necessary dependencies:
bundle install
Setting up the database:
rake db:schema:load
Note that some of you might need to prefix the above command with bundle exec.
One final thing before we proceed to the actual stuff: Create .pryrc in your home directory and fill it in with the editor of your choice:
% touch ~/.pryrc Pry.config.editor = 'vim'
To launch pry through the terminal you simply write:
rails c
and you’re greeted with a pry console:
% rails c Loading development environment (Rails 3.2.16) Frame number: 0/3 [1] sample_app »
Usage and Example
Usage
After you install Pry using the above mentioned install command, you run your program using rescue foo.rb instead of ruby foo.rb. If not, just wrap chunks of your program in Pry::rescue{ }. Any exceptions that are unhandled within that block will be rescued by pry on your behalf.
Whenever pry opens because of an exception you have two choices:
- Hit ctrl-d to let the exception bubble on its way
- Do some debugging.
If you choose the second option, then you can use the full power of pry to fix the problem; and then try-again to verify the fix worked.
Example
For example, lets assume the following piece of code<ref>http://cirw.in/blog/pry-to-the-rescue</ref>:
def find_capitalized(a) a.select do |name| name.chars.first == name.chars.first.upcase end end
Now if you run rescue foo.rb, you would get a output which would look like this:
From: rescue.rb @ line 2 Object#find_capitalized: 2: def find_capitalized(a) 3: a.select do |name| => 4: name.chars.first == name.chars.first.upcase 5: end 6: end NoMethodError: undefined method `chars' for :direction:Symbol from rescue.rb:4:in `block in find_capitalized' [1] pry(main)>
Well, clearly it's gone wrong. Let's move up the stack and see if we can find which code is calling this method with symbols:
[1] pry(main)> up From: rescue.rb @ line 8 Object#extract_people: 8: def extract_people(opts) => 9: name_keys = find_capitalized(opts.keys) 10: 11: name_keys.each_with_object({}) do |name, o| 12: o[name] = opts.delete name 13: end 14: end [2] pry(main)> opts => {"Arthur"=>"Dent", :direction=>:left}
Let's see if there's a better way of implementing find_capitalized:
[3] pry(main)> down [4] pry(main)> name.first NoMethodError: undefined method `first' for :direction from (pry):9:in `block in find_capitalized' [5] pry(main)> name.capitalize => :Direction
Let's edit-method to fix the code, and try-again to verify the fix worked:
[6] pry(main)> edit-method [7] pry(main)> whereami From: rescue.rb @ line 2 Object#find_capitalized: 2: def find_capitalized(a) => 3: a.select do |name| 4: name.capitalize == name 5: end 6: end [8] pry(main)> try-again Arthur Dent moves left
Pry Commands
There are a myriad of pry commands that are useful while debugging. Follwing is the list of some important list of commands that can be used while debugging using Pry<ref>http://www.jackkinsella.ie/2014/06/06/debugging-rails-with-pry-debugger.html</ref><ref>http://cirw.in/blog/pry-to-the-rescue</ref><ref>http://www.sitepoint.com/pry-friends-rails</ref><ref>https://github.com/ConradIrwin/pry-rescue</ref>.
- 1. ls <Object>
- Description: It show all of the available methods that can be called by an object
- 2. _
- Description: Last output
- 3. ? <Object>
- Description: Shows more information (doc) about an object, or method
- 4. cat <File>
- Description: Display the content of a file
- 5. _file_
- Description: Represent the last file Pry touched
- 6. wtf?
- Description: Print the stack trace, same as _ex_.backtrace
- 7. $
- Description: Show source, shortcut for show-source
- 8. edit <Method>
- Description: Open file in $EDITOR, change file are auto reloaded
- 9. <ctrl+r>
- Description: Search history
- 10. _out_
- Description: Array of all outputs values, also _in_
- 11. cd
- Description: Step into an object, change the value of self
- 12. cd ..
- Description: Take out of a level
- 13. binding.pry
- Description: Breakpoint
- 14. edit --ex
- Description: Edit the file where the last exception was thrown
- 15. .<Shell>
- Description: Runs the <Shell> command
- 16. whereami
- Description: Print the context where the debugger is stopped
- 17. ;
- Description: Would mute the return output by Ruby
- 18. play -l
- Description: Execute the line in the current debugging context
The best thing to do is just type help once you've installed pry.
Remote debugging
Remote debugging means you work on your local computer and you want to start and debug a program on another computer, the remote machine. Pry is a great tool for digging into your code and seeing what's going on with tons of great features. However, there are situations where using a standard binding.pry breakpoint will not block your program and allow you to inspect it.A remote session allows you to start instances of Pry in a running program and connect to those instances over a socket. This is particularly useful for using Pry in places not normally possible, such as apps running on the Pow web server. It also opens the door for such exotica as multi-user sessions.
Pry has two plugins that implement remote sessions: 'pry-remote' and the newer and more ambitious 'pry-remote-em'<ref>https://github.com/Mon-Ouie/pry-remote</ref>.
pry-remote: A way to start Pry remotely and to connect to it using DRb. This allows to access the state of the running program from anywhere. Simply replace the normal 'binding.pry' call with 'binding.remote_pry' as the example below illustrates.
We set up the server as follows:
require 'pry-remote' class Foo def initialize(x, y) binding.remote_pry end end Foo.new 10, 20
We can then connect to it using the 'pry-remote' executable.
pry-remote-em: pry-remote-em is a EventMachine-based alternative to pry-remote. It adds user authentication and SSL support along with tab completion and paging. It also allows multiple clients to connect to the same server, and multiple servers to run on the same computer and even within the same process.
require 'pry-remote-em/server'
class Foo def initialize(x, y) binding.remote_pry_em end end EM.run { Foo.new 10, 20 }
Comparison with other popular debugging tools
Pry is a cross-over REPL because it supports some of what Ruby Debugger does with addons and sometimes even built and also supports all of what IRB does with some enhancements such as tab completion, code spacing and syntax highlighting as well as supporting an easy way to tap into an object with an REPL easily. There are also other things like the ability to show the source of a class, a method and source around where you are (if you are in a method)<ref>http://www.infoq.com/articles/ruby-debuggers-survey</ref>
Other debuggers available for Ruby are:
1.Komodo: The Komodo debugger is a tool for analyzing programs on a line-by-line basis, monitoring and altering variables, and watching output as it is generated.
2. Eclipse's EclipseMonkey extension allows scripts written in JRuby. As these scripts run inside the same JVM as the Eclipse IDE, it's possible to access the Debugger instances and control it.
3. SapphireSteel's Ruby in Steel IDE is also built using native code to implement the functionality, complete with using Ruby hooks to get notified about events such as method invocations etc.
4. Aptana RadRails: Aptana RadRails sets breakpoints, inspect variables, control execution. The integrated Ruby & Rails debugger helps you squash the bugs.
5. RubyMine: RubyMine has an excellent step-through debugger which lets you set breakpoints in your code and trace through the program. It provides a useful feature for exploring all of the metaprogramming going on behind the scenes in Ruby on Rails.
See also
References
<references />