CSC/ECE 517 Fall 2014/ch1a 1 rm
Play Framework
Play is modern web framework that provides support for writing scalable applications in either Java or Scala. It is an open source web framework and follows model–view–controller MVC architectural pattern. It mainly focuses on being stateless and non-blocking I/O which is one of the main drawbacks among its competitors like Tomcat, Spring MVC etc. Unlike these, Play’s approach is not threaded, it is a framework that is based on event model. This support for a full HTTP asynchronous programming model, makes Play an ideal framework for writing highly-distributed systems while maintaining performance, reliability and developer productivity.
Play is built on Akka(Actor based model), providing predictable and minimal resource consumption for highly scalable applications. It used for handling highly concurrent systems and allows to develop applications in both Java and Scala.<ref> http://www.playframework.com </ref>
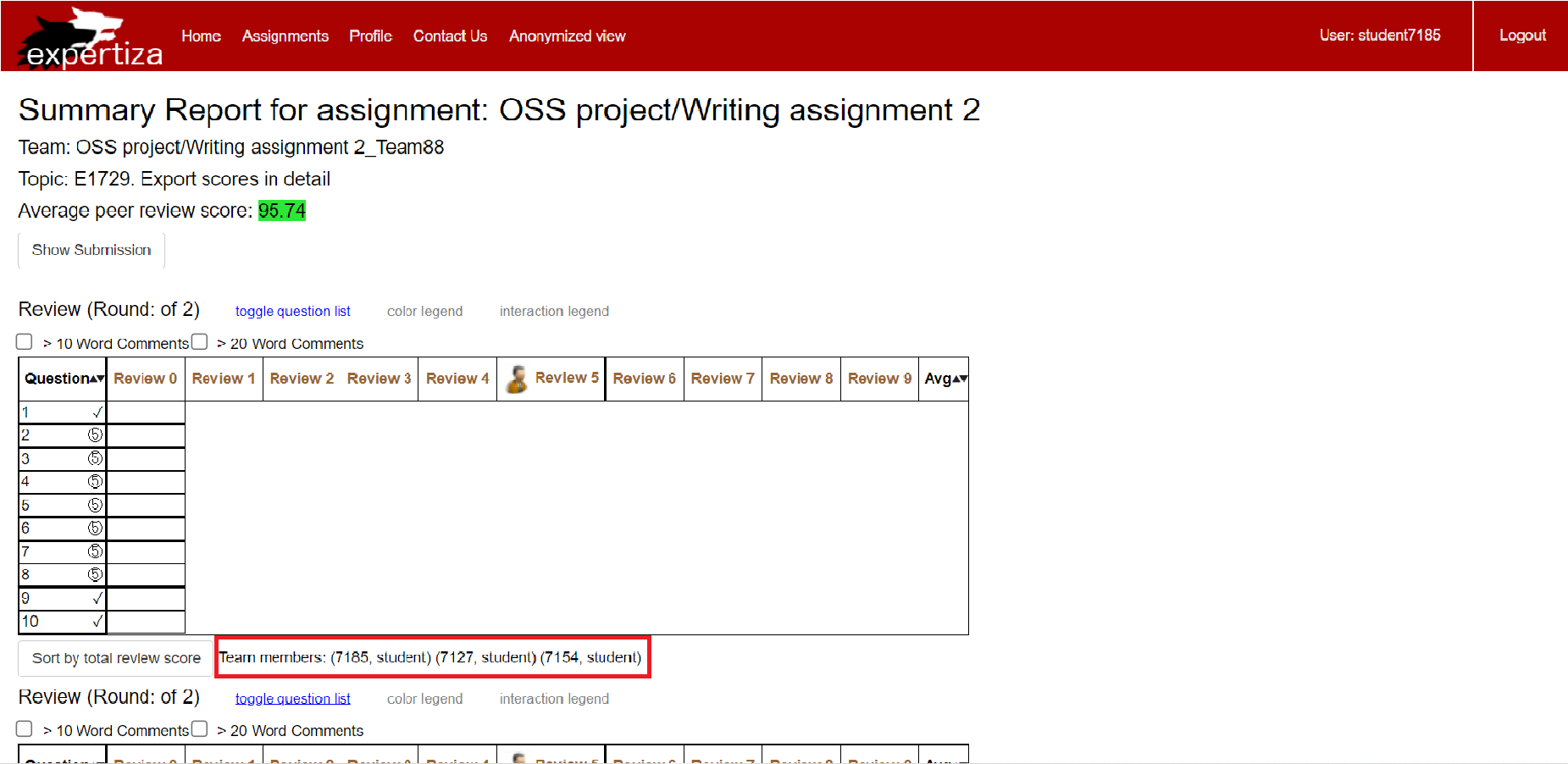
History
Play Framework development started back in 2007 at Zenexity but it’s first release as a open source framework was available not available until 2009. In May 2008 the first published code for 1.0 appeared on Launchpad. This was followed by a full 1.0 release in October 2009.
Play 1.1 <ref name="history">http://en.wikipedia.org/wiki/Play_framework#History</ref> was released in November 2010 after a move from Launchpad to GitHub. It included a migration from Apache MINA to JBoss Netty, Scala support, native GlassFishcontainer, an asynchronous web services library, OAuth support, HTTPS support and other features.
Play 1.2 <ref name="history"/> was released in April 2011. It included dependency management with Apache Ivy, support for WebSocket, integrated database migration (reversion is not implemented yet), a switch to the H2 database and other features.
Play 2.0 was released on March 13, 2012 in conjunction with Typesafe Stack 2.0.
Play 2.1 <ref name="history"/> was released on February 6, 2013, upgraded to Scala 2.10 and introduced, among other new features, modularisation, a new JSON API, filters and RequireJS support.
Play 2.2 <ref name="history"/> was released on September 20, 2013. Upgraded support for SBT to 0.13, better support for buffering, built in support for gzip and new stage and dist tasks with support for native packaging on several platforms such as OS X (DMG), Linux (RPM, DEB), and Windows (MSI) as well as zip files.
Play 2.3<ref>https://www.playframework.com/documentation/2.3.x/Highlights23</ref> has updated to include Activator, improved build environment(sbt), compatibilty with Java 8. This version of Play has been built on multiple version of Scala both 2.10 and 2.11. The WS client library has been refactored into its own library so that Play can have multiple WSClient objects, rather than only using the WS singleton. A method to use actors for handling websocket interactions has been incorporated for both Java and Scala. Anorm ( a simple data access layer that uses plain SQL to interact with the database and provides an API to parse and transform the resulting datasets) has been updated to include type safety, option parsing, error handling.
Development with Play
Let us see the features offered by Play from a developers perspective, who wants to develop a web application in Play framework.
Installing Play
To install Play framework, download the binaries from download page and extract the contents to any folder. Add the extracted folder to path variable<ref>https://www.playframework.com/documentation/2.3.x/Installing </ref> and verify whether you were able to execute the below command :
activator ui
This starts the play server and if everything goes well, you should see a default play application running at http: //127.0.0.1:8888/home .
Creating a new project
To create a new project just type the below command and Play will take care of creating directory structure for your app with a standard layout.
activator new <app name>
If you are wondering what folders were created and what they imply, here is a brief explanation to get you started :
- app folder: It contains the main part of the application,i.e.,models, views, controllers. All the source code is present here.
- conf folder: It will contain all the configuration files especially the application.conf, the routes file and the messages file used for Internationalization(a way of adopting your application to different languages for different regions). The main entry point of the application is conf/routes file, it will contain the list of URL’s that are accessible in a application.
- lib folder: It is used for extending libraries.
- public: It used for all static files like JavaScript files, style sheets and images directories, resources which are available publicly.
- test folder: All the application tests are written in this folder, JUnit for functional testing and Selenium tests for Web application testing.
There won’t be any predefined folder to store Java .class files, as Play doesn’t need one. It internally uses eclipse compiler and compiles Java classes on the fly. This gives the ability to reflect the changes made in Java files, without having to compile them again. Another advantage of having an inbuilt compiler is that Play will create a better error report by showing the point of failure <ref>http://stackoverflow.com/questions/11704951/play-framework-no-need-to-compile</ref>.
How to run an application?
Navigate to the app directory and execute below command :
activator run
Your application will be loaded and a web server is started at 9000 port. Application can be accessed at http://localhost:9000/
Similar to Rails, Play also uses config/routes file to map the request URL to the respective controller method. A sample entry looks like this :
Get / Users.index
The above line invokes index() method in Users controller when you access the application home page.
You can either use a text editor to make code changes or even there is a support for IDEs like Netbeans and Eclipse in Play. If you want to set up a Java IDE, please check this page.
Database support
Play comes with an inbuilt Database called HSQLDB<ref>http://en.wikipedia.org/wiki/HSQLDB</ref>, this is an in-memory database and if you want to use any other persisting databases like Oracle, Postgres etc, you can add those configs in conf/application.conf file. Play will connect to the specified database while application startup.
Play uses 'Object Relational Mapper' to persist the model objects to a persistent DB. JPA<ref>http://en.wikipedia.org/wiki/Java_Persistence_API</ref> is a Java specification that defines a standard API for object relational mapping. As implementation of JPA, play uses the well-known Hibernate framework.
Testing
To run a test case, you need to start the application in a special ‘test’ mode. Stop the currently running application, open a command line and type:
activator test
The 'play test' command is almost the same than 'play run', except that it loads a test runner module that allows to run test suite directly from a browser. When you run a play application in test mode, play will automatically switch to the test framework id and load the application.conf file accordingly. Check this page for more information. Open a browser to the http://localhost:9000/@tests URL to see the test runner.
Similar to Rails, one can use Fixtures to load the test data in test. These data files reside in appname/test/ folder.
Play gives you a way to test directly the controller part of the application using JUnit. These are called 'Functional tests'. Basically a functional test calls the play ActionInvoker directly, simulating an HTTP request. So we give an HTTP method, an URI and HTTP parameters. Play then routes the request, invokes the corresponding action and sends you back the filled response. You can then analyze it to check that the response content is like you expected.
Play provides an additional functionality for Selenium tests, which are specifically written for web based applications. The benefit of using Selenium is that it will test your application in your browser and not a built in simulator.
Play Templates
Play has an efficient templating system which allows to dynamically generate HTML, XML, JSON or any text based format document. The template engine uses Groovy as an expression language. A tag system allows you to create reusable functions. Templates are stored in the app/views directory.
This template language is useful in various ways and you can read more about it here
Play Modules
A play application can be assembled from several application modules. This lets you reuse components across several applications or split a large application into several smaller ones. A module is just another play application; however some differences exist in the way resources are loaded for an application module : A module does not have a conf/application.conf file. A module can have a conf/routes file, but these routes will not be loaded automatically. All files are first searched for in the main application path, then in all loaded modules. Everything in a module is optional. You can create a module with the play new command, just like you do for any other application.
To load an external module<ref>https://www.playframework.com/modules</ref>, just declare it in the application.conf file of main application. One of such useful modules in CRUD module. Using CRUD you can easily configure your application to have an administration area where you can add/update/delete any model objects present in your application.
Another such frequently used module is a code coverage module based on the Cobertura tool.
Why Play?
Play is a lightweight framework that offers so many options for Java and Scala developers, without compromising much on productivity. Here are some of the features describing why one should opt for Play:
Developer Friendly
- Change the code wherever like Java classes or Templates and just hit refresh to see your changes.
- Lot of support for testing tools.
Scalable and Efficient
- Play is built on Akka, so it supports non-blocking I/O<ref>http://www.playframework.com/documentation/2.0.4/ScalaAsync</ref>. This means it's very easy and inexpensive to make remote calls in parallel, which is important for high performance apps in a service oriented architecture.
Good Support
- Commercial support is provided by Zenexity and Typesafe.
- Easy cloud hosting options like Stax cloud hosting platform and can be deployed virtually anywhere: inside Servlet containers, as standalone servers, in Google Application Engine etc.
Open source
- Play is a open source project and this enables you to see what happens under the hood.
- Also there is vast support through plugins modules etc which can be reused in building your application.
Built for latest technologies
- Play has extensive NoSql and BigData support.
- Built-in support for REST, JSON/XML handling, non-blocking I/O, WebSockets <ref>http://www.playframework.com/documentation/2.0.4/ScalaWebSockets</ref>, asset compilation (CoffeeScript, less), ORM, NoSQL support, and many.
Notable uses of Play
LinkedIn is the one of the most popular companies that uses play in its production.<ref> https://engineering.linkedin.com/play/play-framework-linkedin</ref> Some of the other websites that use Play are Coursera, Mashape, typesafe, Prenser, PeachDish<ref>http://en.wikipedia.org/wiki/Play_framework#Usage</ref>.
Comparison with other frameworks
Project | Language | Ajax | MVC framework | MVC push-pull | Testing framework(s) | DB migration framework(s) | Security framework(s) | Template framework(s) | Caching framework(s) | Form validation framework(s) | Scaffolding |
Ruby on Rails | Ruby | Prototype, script.aculo.us, jQuery | Yes (ActiveRecord, Action Pack) | Push | Unit Tests, Functional Tests and Integration Tests | Yes | Plug-in | Yes | Yes | Yes | Yes |
CakePHP | PHP >= 5.2 | Prototype, script.aculo.us, jQuery, MooTools | Yes | Push | Unit tests, object mocking, fixtures, code coverage, memory analysis with SimpleTest and XDebug PHPUnit (cakephp 2.0) | Yes, CakePHP Schema Shell by default, and some others | ACL-based | Themes, layouts, views, elements | Memcache, Redis, XCache, APC, File | Validation, security | Yes |
Django | Python | Yes | Yes | Push | unittest, test client, LiveServerTestCase | Provided by South(http://south.aeracode.org/), Django Evolution | ACL-based | Django Template Language | Cache Framework | Django Forms API | No, not by default |
Play | Scala/Java | Yes | Yes | Push-pull | Unit test, Functional test, Selenium | Yes, similar to Ruby on Rails | via Core Security module | Yes | Yes | Server-side validation | Yes |
References
<references/>