CSC/ECE 517 Fall 2014/ch1a 11 ap
Automated Refactoring Techniques for AppCode
Introduction
AppCode<ref>http://en.wikipedia.org/wiki/AppCode</ref> is an Integrated Development Environment (IDE) for Objective-C,C, C++, and JavaScript development built on JetBrains’ IntelliJ IDEA platform, which is built on Java. Users can extend its abilities by either installing plug-ins created for IntelliJ Platform, or they can write their own plug-ins.
Code refactoring<ref>http://en.wikipedia.org/wiki/Code_refactoring </ref> is the process of restructuring existing computer code – changing the factoring – without changing its external behavior. Many a times, we face the need to change the name of a variable in our code- mostly because we find another one better suited for its functionality. Doing this manually can be time consuming & often leads to many mistakes. That is why, many new IDEs (Integrated Development Environments) come with inbuilt functions for refactoring. As you read further, you will know that refactoring is not limited to changing variable name. There are many things IDEs allow programmers to do to edit their code. These changes are not logical, in the sense that they do not make any change to functionality or behavior of the program; but are needed to improve code in terms of understanding & user friendliness.
Automated refactoring<ref>http://stackoverflow.com/questions/tagged/automated-refactoring </ref> refers to the restructuring of source code (see refactoring) where a computer program does the structure modification, usually guided by user input. Many IDEs have automated refactoring capabilities that significantly reduce the possibility of error when restructuring code.
Background
"By continuously improving the design of code, we make it easier and easier to work with. This is in sharp contrast to what typically happens: little refactoring and a great deal of attention paid to expediently adding new features. If you get into the hygienic habit of refactoring continuously, you'll find that it is easier to extend and maintain code." —Joshua Kerievsky, Refactoring to Patterns<ref>http://martinfowler.com/books/r2p.html</ref>
Refactoring improves nonfunctional attributes of the software. Advantages include improved code readability and reduced complexity to improve source code maintainability, and create a more expressive internal architecture or object model to improve extensibility. To help programmers easily improve code design as it evolves over time, AppCode provides a solid set of reliable code refactorings. These include vital ones like Rename, Extract Method, Introduce Variable and Inline, as well as more powerful ones, e.g. Extract Block Parameter/Protocol/Category/Superclass, Change Signature, Convert Method to Function and back, Pull Members Up/Push Members Down, and others. First public preview version of AppCode became available in April 2011. The latest stable release is version 3.0 and is available at JetBrains’ official web site.
Features of AppCode <ref>http://www.jetbrains.com/objc/features/</ref>
1. Finest coding assistance The context-aware editor in AppCode gives you precise code completion choices due to deep understanding of the code structure an therefore avoids the need for unnecessary typing by generating code and also minimizes the routine tasks. AppCode automatically imports a class if it is not imported by the programmer in the code. Parameter Info(ctrl+P) assists the programmer in determining what exact parameters need to be passed to a method or function. Code reordering allows you to order your code. For example, to move block of code up or down, the following commands can be used:Cmd+Shift+Up/Down and for lines of code: Alt+Shift+Up/Down. Such assistance allows user to concentrate more on the logic of program than its structure.
2. Reliable refactorings AppCode provides safe, accurate and reliable refactorings to modify and improve your code at any time. AppCode can be used to revive old code base, or brush up the project structure. Various refactoring techniques mentioned below make it easy to refactor code.
3. Fast project navigation AppCode provides a set of navigation features to search through any code no matter how tangled it is. Navigations from a method to its declaration or any of its usages, through classes hierarchy or from one file to another are very easy to follow. The programmer can jump to any file, class, or symbol in a project in no time, or see all real usages of a symbol, not just text matches. The Smart code editor on the right side has tabs for easy navigation between open files. Navigation bar above the editor additionally allows to quickly run, debug, test or profile an application.
4. Code quality tracking On-the-fly code quality analysis for Objective-C, C, C++, JavaScript, CSS, HTML, XML and Xpath is found in AppCode. AppCode prevents potential errors, and gives hints where your code can be improved. Moreover, it comes with integrated Clang Static Analyzer.
5. Powerful debugger Programmers can fine-tune apps or unit tests using a convenient debugger with flexible breakpoints, watches, frames view and evaluate expressions. With the help of conditional breakpoints in AppCode, there is more control over the debugging process, and an option to log an evaluated message when a breakpoint is hit.
6. Seamless integrations
AppCode seamlessly integrates with the most popular version control systems, like Git, Mercurial, Perforce, etc.; Kiwi testing framework, Dash and Ingredients documentation tools, a number of issue trackers and provides 100% interoperability with Xcode. AppCode is also integrated with Reveal , a fantastic tool from Itty Bitty Apps that helps debug iOS applications. Using the tool the programmer can inspect view elements and hierarchies of an iOS apps in real time, use the 2D and 3D visualization possibilities, and apply the view's editing on the fly, which is a convenient way to tweak the interface without recompiling.

Refactoring Techniques in AppCode: <ref>http://www.integralist.co.uk/posts/refactoring-techniques/</ref> <ref>http://blog.bonkowski.net/blog/2013/02/10/refactoring-in-appcode/</ref>
Automated Techniques used by AppCode are listed below:
1. Rename
By using this technique, one can rename a file, class, method, property, ivar, local variable, and so on. In the file or project view, the shortcut triggers renaming a file or a class. To just rename a file (such as a plist or an image), AppCode will rename the file, and also change where it is referenced. If you are renaming a class, both the header, and the implementation of the class is renamed, and the references of the files in the project file. If you select Rename when the cursor is on a class name used by the class, the selected class is renamed. If the rename is triggered from inside the source file, the name of the class, variable, and so on, is entering a “edit mode,” like “Edit all in Scope,” in Xcode. The difference is that while in Xcode, only the names inside the current class were renamed.
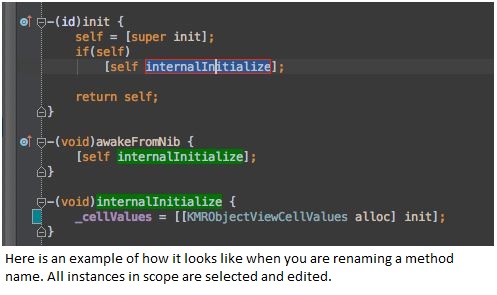
2. Change Signature
Often, a programmer needs to alter a method signature. This can be to include an additional argument, or maybe the argument is not needed anymore, or even the order of the arguments is not right. If the method is called from several places, this can be a time-consuming and error prone task. By using AppCode, this is done in a simple and safe way. This is a really powerful feature in AppCode. You can also change the return type, and even convert a method to a function or a block, or vice versa.
3. Convert
Converting a method to a function or a block can also be done by using one of the Convert to menu items. In addition to these, you also have Convert to Property and Convert to Instance Variable.
4. Move
Sometimes, a method may be defined in the wrong class. The Move refactoring technique allows you to move a property, variables and methods from one class to another. If the target class does not exist, you get a warning that tells you that. Once the method is moved, the previous parameters that were being sent to the method need to be cleaned up and it should be checked whether additional data needs to be passed over now via the method invocation.
5. Copy
In the project browser, when selecting a header, or an implementation file, or when inside one of these files, F5 will prompt you to enter the name of the new copy of the files, and where to copy them. When accepting, the class name inside the source files is changed as well.
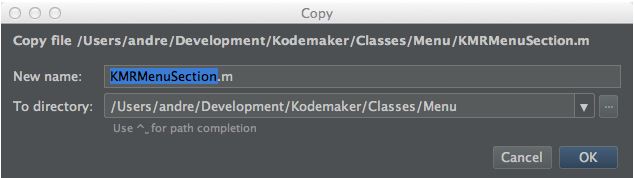
6. Safe Delete
Some implementations might later found to be redundant or irrelevant in the code. Removing such implementations can be tricky. Manual deletions can lead to many misses & references to undefined data. That is where AppCode comes in handy. While deleting a class or a file, the programmer checks if that class or file is being used anywhere else in the program. Using Safe Delete, the programmer can be warned of any such multiple usages through a warning message. The search is also extended to comments and strings.
7. Extract
To quickly move code around in a full swing of refactoring, one can extract an expression to a variable or extract a full code block into a new method, and add a parameter to it using Introduce Parameter refactoring. This is a much mode tedious job than one can imagine and programmer can often lose the link of what needs to be changed while manipulating code for this.
With Ctrl+T, AppCode shows what refactorings are suitable for the current context. The new Move refactoring allows you to move any class or member, or even a top level element, say a namespace through your project. Not only will AppCode move an element, but it will update the references, automatically manage imports and escalate visibility if needed. These might look as far fetched cases to a beginner; but when operating on a complex application that is being used for decades and has gone through many changes and additions over the years, these advanced refactoring techniques pose much more need than anticipated.
There are several Extract factoring’s, and here is a short description of each:
1. Extract Method This is one of the most frequently used refactoring. Let say a method has too much responsibility, and you want to move some of the logic into a new method. AppCode generates a new method for you, including necessary parameters.
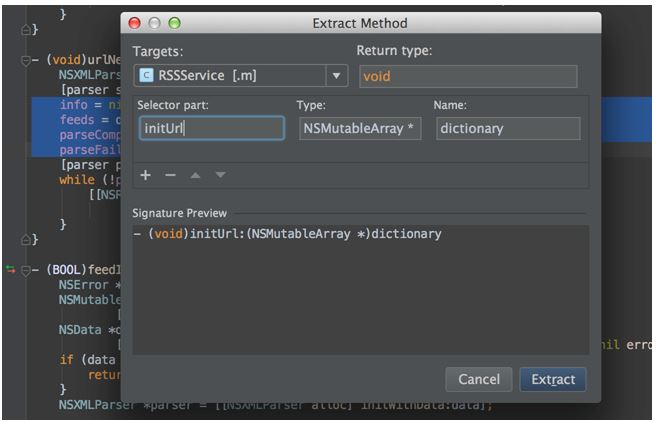
2. Extract Variable If there are some hard coded values in the code and we later feel the need to generalize the concept to a set of values, we would need to "Extract a variable". Here, AppCode will declare a variable above where it’s used, with the correct type, and the name of the new variable selected. To change the proposed name, just begin to type the name of the variable. If no value or expression is explicitly selected, AppCode gives you a suggestion of what to base the value of the introduced value from.
3. Extract Constant This refactoring is similar to Extract Variable, but this introduces a constant instead. If no actual value is selected, AppCode gives you an opportunity to select what the constant is based on from values and expressions in scope. When the constant is selected, AppCode asks for you if you would like to replace only this one, or all similar values found in the current file.
4. Extract Parameter This can be used if you are creating a variable inside a method, but you would like it to be passed as a parameter.
5. Extract Property By using this property on a variable, AppCode shows a dialog where the declaration of the property is shown, togheter with a checkbox. If you check the checkbox, the property is generated in a private category. If not, the property is declared in the header.
6. Extract Instance Variable By using this on a local variable, AppCode changes it into an ivar, but with the option to generate a property for the variable if the checkbox is selected. You can also select to declare the ivar in the interface (which you don’t want to do).
7. Extract Define Extracts a macro from the selected value or expression
8. Extract Typedef As the name suggests, it creates a typdef from the selected type.
9. Extract Block Parameter By using Extract Block Parameter you select a chunk of code. A block declaration based on the selected code is created, and the signature of the method is changed to take a block of this type. Finally, the existing usages of this method are changed so that the extracted block is passed as a parameter.
10. Extract Superclass To create a superclass from an existing class, the easiest way to accomplish this is to select the Extract Superclass. This refactoring does not have a default keyboard shortcut (You can create one yourself). You will be presented with a dialog where methods, properties, and ivars are shown in a list with checkboxes, and an input field where you type the name of the new superclass. You check the name of what you want to move into the new superclass. If one of the selected methods has dependencies to other methods, properties, or ivars, you get a warning, telling you about the problem.
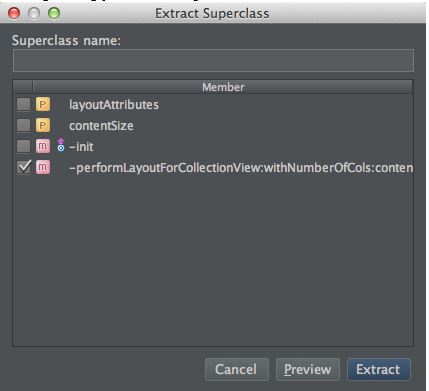
11. Extract Subclass Here you create a subclass from a superclass. You select which methods, ivars and properties you want to take with you into the new subclass.
12. Extract Protocol and Category Similar to the two above, except you are extracting protocols and categories.

8. Inline
If you want to inline a method, AppCode will move the implementation of the method into where it’s called from. If the method is called from more than one place, AppCode prompts you and asks for “Do you want to inline ‘n’ usages of method ‘methodName’? You options are: cancel the refactoring, execute the operation or view usages of the method you have selected to inline. If you select the latter, a list of usages is shown, and while navigating in this list, you can exclude the calls you don’t want to inline
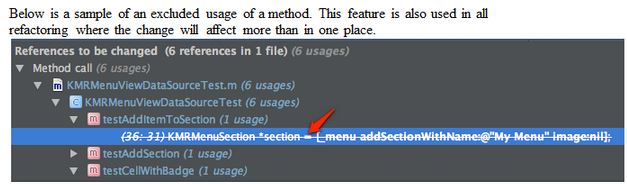
9. Pull Up Method
We may end up creating methods in subclasses that essentially do the same thing. They can often be "pulled up" to their superclass. In this method of refactoring, such duplicated code across two separate classes is "pulled" into a super class so that we DRY ([Don't Repeat Yourself]) out the code and use it in multiple places without duplication (i.e, changes in future only have to happen in one place).
10. Push Down Method
This is exact opposite of pull up. This is where a method is defined in superclass but not implemented in the same way in its subclasses. Then, AppCode allows us to "Push Down" such method to its subclasses and we can write different code in each.
Comparison: Xcode vs AppCode <ref>http://codesheriff.blogspot.com/2012/02/xcode-vs-appcode.html</ref>
Another refactoring tool that is widely used and well known is Xcode. Xcode allows 6 types of refactoring techniques including 'create superclass', 'pull up','push down'. This is in contrast to the vast variety of techniques offered by AppCode. AppCode also provides keyboard shortcuts for faster refactoring. With each release, AppCode keeps getting better to suit the needs of programmers.
Xcode | AppCode |
Xcode offers only six refactoring commands such as 'create superclass', 'pull up','push down' without any keyboard shortcuts. | AppCode offers several techniques that are safe and accurate with almost every technique having a built-in keyboard shortcut by default. |
Quick Fix in Xcode is very basic and limited. | AppCode has much more powerful Quick Fix features with easy to use keyboard shortcuts. |
For code generation or completion, the method-name-completion mode needs to be selected and browsed for options. | AppCode provides a better set of options to override/implement a method. |
In Xcode, a single specific test cannot be tested for, all tests must run together. | In AppCode, a single test can be run and if it fails, a log is provided for the failing run. |
Xcode has very simplistic git integration and is therefor easy to use and understand. | AppCode's git integration is more robust and can be done from a single place. |
References
<references/>
Further Reading
1. Refactoring: Improving the design of existing Code By Martin Fawler
2. A paper on "Why Don’t People Use Refactoring Tools?"