CSC/ECE 517 Fall 2013/ch1 1w27 ma
MVC Architecture Structure In Ruby on Rails
The aim of this wiki is to explain the explain the different components of a Rails application. It is directed towards a novice user who can read and get an overview, not the details of any particular kind of component. In depth discussion links are made available in the further reading section.
Introduction
Ruby on Rails (RoR) is open source web framework that is focused on productivity and enforces agile web development. Rails framework leverages the features of the Ruby language. Ruby is a dynamically typed, object-oriented, scripting language that was designed to have an elegant syntax and made as human readable as possible.
The Ruby on Rails framework was designed for database-backed web applications. In order to make the development process faster, Ruby on Rails uses conventions and assumptions which eliminates configuration code and increases productivity. Many tasks like email management, object-database mappers, file structures, code generation etc are built in.
What is MVC
MVC stands for Model-View-Controller and is a design pattern and was developed in 1979 by Trygve Reenskaug. MVC dictates that the system be split into three distinct parts, a Model, View and Controller. These correspond to the business logic, the presentation logic and the application flow respectively. This approach organizes the code into separate components, thereby achieving separation of concerns and facilitating maintainability.
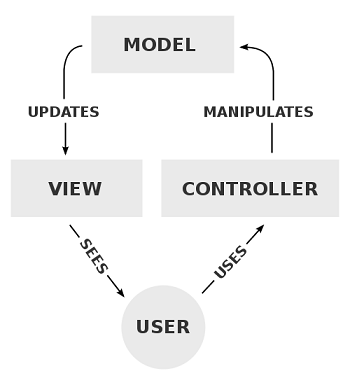
Model
- The Model generally contains the data for the application and is usually linked to a database back-end. It contains the application state, validation and also the business logic. A central component of Rails is the class ActiveRecord, which maps relational tables to Ruby objects and thereby to the data manipulated by controllers and shown in views. Models in Rails inherit this class.
- Rails provides tools to implement the CRUD functionality with zero-configuration. CRUD allows creating, reading, updating and deleting records from the database through ruby objects. An model object in Rails represents a row in a database table. Additionally, Rails provides advance search capabilities and the ability to create relationships or associations between models. The model has no knowledge of the user interfaces [the view].
View
- The view refers to the interface that is presented to the end-user and includes HTML, CSS, XML, Javascript, JSON. The view does not do any processing, but simply acts as the presentation layer , displaying the application data. Views in Rails consist of .erb files that combines pure HTML with embedded Ruby code.
Controller
- The controller receives events from the outside world [ or through some view]. It is the facility within the application that directs traffic. It interacts with the model and redirects to the appropriate view. The controller queries the models for specific data, and also organizes that data (searching, sorting, massaging it) into a form that fits the needs of a given view. In Rails, controllers should descend from ApplicationController which itself descends from ActionController::Base
Thus the decoupled nature of MVC gives us more flexibility and allows us to easily maintain our application. For example, we can have multiple possible views for the same model and can switch between them if required. Also, view developers are only concerned with the model structure and need not know the underlying architecture,
How Rails MVC Works
MVC Architecture working can be explained as follows: The model, view and controller are referred to as Active Record, Action View and Action Controller respectively here.
- 1. The browser makes a request, such as http://myblog.com/videos/show/10
- 2. The web server receives the request. It uses routes to find out which controller to use. The default route pattern is /controller/action/id as defined in config/routes.rb. In this case, it is the video controller, method show, id 10. Controllers do the work of parsing user requests, data submissions, cookies, sessions. In this case, the show method in the video controller knows it needs to lookup a video. It asks the model to get video 10, and will eventually display it to the user.
- 3.The Model(ActiveRecord) is implemented in ActiveRecord library which provides an interface and binding between the tables in a relational database and the Ruby program code that manipulates database records.
- 4. In this case, the model retrieves video 10 from the database.
- 5. Model sends the retrieved data ie video 10 in this case to the controller.
- 6. The Views(ActionView) subsystem is implemented in ActionView library which is an Embedded Ruby (ERb) based system for defining presentation templates for data presentation. In our example, the controller gives video 10 to the show view.
- 7. The show view generates the HTML: divs, tables, text, descriptions, footers, etc and returns it to the controller.
- 8. The controller returns the response body (HTML, XML, etc.) & metadata (caching headers, redirects) to the server. The controller subsystem is implemented in ActionController which is a data broker sitting between ActiveRecord (the database interface) and ActionView (the presentation engine). The server combines the raw data into a proper HTTP response and sends it to the user's browser.
Directory Structure & Components
The primary components of Rails are
- Application components like model, view, controller, helpers
- Environment & Routing Configuration
- Database Migration Components
- Test classes
Now we look at how these components are organised into different directories. The default directory structure of a generated Rails application is as follows:
|-- app | |-- assets | | |-- images | | |-- javascripts | | `-- stylesheets | |-- controllers | |-- helpers | |-- mailers | |-- models | `-- views | `-- layouts |-- config | |-- environments | |-- initializers | `-- locales |-- db |-- doc |-- lib | |-- assets | `-- tasks |-- log |-- public |-- script |-- test | |-- fixtures | |-- functional | |-- integration | |-- performance | `-- unit |-- tmp | `-- cache | `-- assets `-- vendor |-- assets | |-- javascripts | `-- stylesheets `-- plugins
app/
This folder organizes the different application components. These include view (views and helpers), controller (controllers), and the backend business logic (models). There are the following sub-directories
- app/controllers: This is the sub-directory where Rails looks for the controller classes.
- app/models: The directory for the models
- app/view: The views subdirectory holds the display templates. The application data is used to fill these templates. The resultant HTML response is sent to the user's browser.
- app/view/layouts: Layouts directory contains the template files for layouts to be used with views. Examples of layouts are the common header/footer for the views.
- app/mailers: This directory contains all the mailer files used to send emails. The ActionMailer module in Rails can handle simple text or complex rich-format emails. Also it has common tasks built-in, such as, sending out forgotten passwords, welcome messages, and fulfilling any other written-communication’s need.
- app/helpers: These are the helper classes used to assist the MVC classes. Helpers are the only methods one can access in the views, other than instance methods for an instance you have access to. Helpers make it possible to keep rest of the MVC code uncluttered.
- app/assets: The assets folder has sub-directories to store images, javascripts and stylesheets. Rails has an asset pipeline which concatenates and minifies JavaScript and CSS assets. It also adds the ability to write these assets in other languages such as CoffeeScript, Sass and ERB.
config/
This directory contains the configuration code that the application needs. This includes database configuration, environment structure , routing of incoming web requests.
- config/environments: It is possible to execute some environment specific code in Rails. Rails automatically detects your current environment [development, test or production] and executes the environment file in this directory. For example, it will run config/environments/development.rb for the development environment. Thus we can tailor the behavior of the three Rails environments for test, development, and deployment with files in the environments directory.
- config/initializers: Any Ruby file stored in this directory is considered to be an initializer. It holds configuration settings that should be made after all of the frameworks and gems are loaded. Initializers can be further organised into sub-directories. If there are any ordering dependencies for the initializers,we can control the load order by naming. The initializers are run in alphabetical order.
- routes.rb: The routing system in Rails is the system that examines the URL of an incoming request and determines what action should be taken by the application. Thus it recognizes URLs and dispatches them to a controller's action. The routes.rb file contains the rules .
db/
Usually, your Rails application will have model objects that access relational database tables. You can manage the relational database with scripts you create and place in this directory.
- db/migrate: Has all the migrations that you created. Migrations are a convenient way to alter your database schema over time in a consistent and easy way. They use a Ruby DSL so that you don't have to write SQL by hand, allowing your schema and changes to be database independent.
- schema.rb: It documents the final current state of the database schema. Often, especially when you have more than a couple of migrations, it's hard to deduct the schema just from the migrations alone. It is used by the tests to populate the database schema.
- seeds.rb: The purpose of seed data is to bootstrap your database. Thus in this file, we initialize the database with default values. For example, things like administrative accounts, or other data that's necessary to run your application for the first time. One shouldn't add anything to a seeds.rb file that isn't necessary to bootstrap your database or its relations
test/
The tests you write and those Rails creates for you all go here. You'll see a sub-directory for unit tests (unit), fixtures (fixtures), and functional tests (functional). There are the following sub-directories
- test/unit: Unit testing is a testing technique to validate that individual “units” of code are working properly. A “unit” refers to the smallest testable part of the code. When unit testing, we are not interested in how all the units fit together, just in make sure that each unit is functioning properly on its own.
- test/fixtures: Fixtures are a way of organizing test data. Fixtures allow you to populate your testing database with predefined data before your tests run. Fixtures are database independent written in YAML. There is one file per model. When you run 'rails generate model' to create a new model, fixture stubs will be automatically created and placed in this directory.
- test/functional: Functional tests live inside of the test/functional directory. These tests are designed to exercise your controllers.
- test/integration: To write an integration test, standard convention is to put them into a test/integration directory. Whereas unit tests exercise models and functional tests focus on single actions within a specific controller, integration tests are meant to test complete workflows, that likely step through multiple actions across one or more controllers.
- test/performance: Rails performance tests are a special type of integration tests, designed for bench-marking and profiling the test code. With performance tests, you can determine where your application’s memory or speed problems are coming from, and get a more in-depth picture of those problems.
Other Directories
- public: This directory contains static files and compiled assets, such as JavaScript files (public/javascripts), graphics (public/images), stylesheets (public/stylesheets), and HTML files (public). The files in this directory are accessible by anyone visiting the site, irrespective of authentication. Error pages (404) are usually stored here
- tmp: Temporary files are stored here.
- vendor: This folder contains plugins, gems & libraries provided by third-party vendors. Example: database utilities library
- script: This directory holds scripts to launch and manage the various tools that you'll use with Rails.
- lib: For library modules.
- log: Error logs for the server (server.log) and each Rails environment (development.log, test.log, and production.log).
- doc: Rails can generate the documentation for your code automatically, if you provide comments in a certain expected format in your code. The generated documentation is placed in this directory.
Gemfile
Gems are Ruby software packages available online. The gemfile is a list of all gems that you want to include in the project. The bundler tool uses the gem file to install, update, remove and otherwise manage existing gems.It is possible to group gems in :development, :test, :assets & :production groups and Rails will know when to include the gems.
Rakefile
The Rakefile is similar to Unix Makefile which helps with building, packaging and testing the Rails code. This will be used by rake utility supplied along with Ruby installation.
README
This file contains a basic detail about Rail Application and description of the directory structure explained above
Pitfalls of the Ruby on Rails Architecture
Build on interpreted language
- As Rails is built on the top of the Ruby language, it inherits its goodness and weakness as well. Ruby is totally object oriented and a dynamic scripting language with an elegant syntax. As it is an interpreted language it is slower than other languages that are compiled like Java or C++. In most cases, this difference in speed is not a problem but the performance starts to degrade when the web application needs to scale to millions of concurrent users.
Not suited for high concurrency
- The Ruby language is not suited for high concurrency applications, because it is not optimized for speed computing. Ruby was designed to be used for rapid development. It does not have good thread support and has trouble dealing with long-lived processes. Another shortcoming is that Ruby’s garbage collector cannot be compared to that of Java. Thus each process requires much more memory.
Deployment concerns
- Although not an architectural flaw, a web application on Ruby on Rails could be harder to deploy than sites that are using more common technologies, such as PHP. That’s because not all the hosting providers support Rails, but the support for Rails is increasing.
Scalability concerns
- Often there are talks about scalability issues in Rails. Developers claim that when dealing with large scale application, the ORM layer turns out to be a bottleneck. As stated above, the lack of good thread support is also a contributing factor. Caching can mitigate some of the speed concerns.
Decision of convention over configuration
- The Rails architecture sacrifices some flexibility in configuration to increase productivity based on conventions that are considered best practices. This is referred to as Convention over configuration
There are some types of applications in which Ruby on Rails Architecture is not suitable:
- Sites that depend on large amount of existing code or legacy code or legacy databases.
- Requirements where dozens or hundreds of websites are residing in a single server.
- Very large-scale web applications (millions of concurrent users) that need to deliver high-performance.
References
- [1] Ruby on Rails for web application
- [2] Rails Directory structure
- [3] Ruby on Rails Tutorial
- [4] Routing Demytified
- [5] What-are-the-benefits-of-MVC
- [6] Ruby-on-rails-architectural-design
- [7] Testing in Rails
- [8] Database Migrations
<references />