CSC/ECE 517 Fall 2010/ch1 S10 PH
GUI Toolkits for Ruby
While starting to build a new desktop or commercial application, we all have to make a very important decision regarding which platform and GUI toolkit to use from a pool of so many available. In very simple words, a Graphical User Interface (GUI) toolkit is the set of API's (Application Programming Interface) that produces the graphical user interface your users will interact with. There are a number of factors to consider when choosing the toolkit which are discussed in the sections below. Different toolkit support different platforms (Linux, Windows, OSX) and have different features such as looks,accessibility and layout engines(how it is rendered on a web browser).We will see all these considerations with respect to Ruby in more details in the sections below.
Peek into the Ruby Language
Ruby is an Object-Oriented Programming language. It serves as a very good platform to write low-level scripts for system administration tasks and for developing plain end-user applications rapidly. Using Ruby definitely avoids the time consuming 'code-compile-test' cycle which is normal in traditional programming.
Despite all these characteristics, Ruby's most usable implementation was the open source interpreter till very recently. This was because the way Ruby was designed,Ruby did not have very interactive capability other than just accepting text inputs on a console. This would not have gone very far since it is very evident to everyone that graphical user interfaces are a very important for modern end-user applications.As a result, at the onset of year 2009, Ruby was still not well known for being used for developing regular or commercial desktop applications. A possible reason was identified as a lack of good GUI-related libraries.
Overview
Ruby does have something that comes packaged with its standard library and enables users to develop GUI for desktop applications. This 'something' is called Tk. To use Tk for GUI development, you have to install Tk and its interface to connect with Ruby(Ruby binding). But the problem is that it is considered messy,ugly and the API is considered to be from yesteryears.With time,things evolved and today we have the developments of number of third party libraries like FxRuby,WxRuby,QtRuby,GTK-Ruby,Shoes and Swing which give Ruby graphical user interaction (GUI).These libraries give Ruby the user interface elements like text boxes to key in data, buttons to perform certain operation and window displays. More recent alternate implementations of Ruby have their own GUI facilities, such as Cocoa for MacRuby and Swing for JRuby. These toolkits are not really brand new but some like FxRuby, wxRuby, ruby-GNOME2 and RubyQt are simply wrappers for toolkits written in C or C++. Shoes is a graphical library is implemented for Ruby alone.
All the toolkits have some common features but they also differ in many aspects. There is no “best” toolkit in abstract terms.The usefulness of a library is only really tested by trying to use it to perform a specific task. Similarly, the toolkit shortcomings may only become apparent after a using it in substantial and a purposeful way.
Ruby's popularity has double folded in recent years.It remains a small language when compared to C, C++ and Java and hence it is an open question whether having a large number of libraries that more or less provide similar features is an optimal outcome.
Tk
The standard graphical user interface (GUI) for Ruby is Tk. It was initially developed for the Tcl scripting language by John Ousterhout. Tk is known for its widgets hierarchy. There's one root widget which can contain other widgets inside it. Tk applications really do have a good look-and-feel.
To develop an app using Tk in Ruby, we first need to create widgets and then include them in our GUI. Events associated with these widgets are then bound to a method. Tk is mostly used to create simple interfaces because of the nested hierarchy and sometimes trying to create complex interfaces can get troublesome. Tk is best used for smaller GUI needs.
Installation & getting started
Ruby installer comes with Tk associations but user needs to download and install ActiveTcl in order to run a code written using Tk GUI Toolkit. If you have Ruby and ActiveTcl installed on your system then any editor can be used to write a code including a Tk Library
Running Sample Application
To create the above shown GUI, open any basic editor (Notepad++ recommended) and type in the below code of lines. As mentioned above we first start the program by creating a root.
require 'tk' # include modules root = TkRoot.new { title "Sample Application" } # Title as root TkButton.new(root) { # create a button text 'Click this!' # text on the button pack { padx 35 ; pady 35; side 'left' } # setting the properties } Tk.mainloop # run the app's mainloop
The above written sample code is simple to understand. We first include the Tk module in Ruby. Then a root widget(a window in this case) is created and is given the title 'Sample Application'. A button is then created in the root widget and is given the title 'Click this'. Finally using pack the properties are set for defining size, alignment etc.
Pros & Cons
Pros -
- Cross platform
Cons -
- Badly documented
- Not elegant
- Widgets are non-native
Projects developed in Tk
FxRuby
FxRuby is a based on Fox toolkit which is a GUI toolkit written in C++. Using FxRuby one can create powerful cross platform GUI interfaces for Ruby applications. Since Fox toolkit is known for its robust C++ implementation, applications develop in FxRuby are highly optimized and have very good performance.
Look and feel of the window apps built with FxRuby stays native to the platform. FxRuby can also be used to create professional business apps.
Installation & getting started
FxRuby is very simple to install on any platform. Once you install Ruby with the Ruby installer, FxRuby can be downloaded and install using Ruby gems which comes along with the Ruby. Procedure to install FxRuby -
- Install Ruby from Ruby installer
- Open Command prompt
- Execute following command -
>>gems install rubygems
Also to get the sample code and the documentation you can install the FxRuby Package from Rubyforge.
Running Sample Application
To get started either you can open any editor and start writing the code or you can also use the integrated features of Eclipse IDE to build the app. Make sure that you load the 'rubygems' library in order to run apps make in FxRuby.
require 'rubygems' # call modules require 'fox16' include Fox app = FXApp.new # Instantiate the app window = FXMainWindow.new(app, "Sample Application") # Create Window with title adding it as a child of app FXButton.new(window, "Click this") # Creating Button and adding it as a child of window app.create # Create an app with the above controls window.show # render the app app.run # run the loop
The above code includes fox and rubygems modules required for fxruby widgets. An instance of FXApp is then created and a window and the button controls are added as its child. The widgets are then bound to the instance.Using the show method of window, the window is rendered and the instance is displayed.
Pros & Cons
Pros -
- Book available for reference
Cons -
- Binary gems are available for Windows, OS X, and Ubuntu Linux but for other platforms,for installing the gem you need to compile native code.
Projects developed in FxRuby
- BeERP -commercial ERP application
- fxtwitter -is a Twitter client
- Discretizer - for creating geometry and mesh for three dimensional objects.
- FreeRIDE -IDE for the Ruby programming language
WxRuby
WxRuby is yet another very stable GUI toolkit for Ruby. Like Tk , it also features native styling of the widgets across platforms and creates quite robust GUI apps. The wxRuby API is very much C++ oriented and the bindings which wxRuby provides doesn't exactly provide an environment of Ruby development. Hence it may get bit difficult for the Ruby developer to understand things in the beginning.
Installation & getting started
The installation of wxRuby is quite similar to that of FxRuby. You can install wxRuby using Ruby gems if the Ruby is already installed on the system. To get it via gems open a console window and type the following command -
>>gems install wxruby
Again like FxRuby you can install wxRuby package from Rubyforge to get the documentation, sample codes etc for wxRuby. The samples which comes with wxRuby are very interesting, you must try out few of them to know the power of wxRuby.
Running Sample Application
require 'rubygems' # call modules require 'wx' class MyApp < Wx::App # Create a class derived by Wx::App def on_init @wxframe = Wx::Frame.new( nil, -1, "Sample Application" ) # Instantiate a Window frame @wxframe.show # Display the above frame end end app = MyApp.new # Instantiate the app app.main_loop # Run the program loop
In the sample application above, rubygems and wx modules are included first. Then a class MyApp is declared which is inherited from base window class of wx module. In the constructor of MyApp a window frame is created and displayed. Now in the main program, instance of app is created and GUI loop is run.
Pros & Cons
Pros -
- Cross platform
- Larger support community
- Windows support
- Ease of installation
- Comprehensive widget set
- Internationalization
- Liberal licence
Cons -
- Not enough documentation
- API is C++ Oriented
- Take time to develop apps
- Does not install properly on latest Ubuntu
Projects developed in wxRuby
- TextPal -is a text editor for blind and visually impaired people
- Zyps- animated game
- wxRuby Plugin for Google Sketch-Up- helps to create and share 3D models
- Albuin- music player
- wxRIDE- IDE for Ruby programming language
QtRuby
Provides Ruby bindings to the Qt toolkit. This is used in the the KDE desktop system.
Installation & getting started
To install QtRuby one need to install cross platform Qt framework available at Trolltech website. If you have Ruby installed, you will only need qtruby installer if you want to install it in Windows.
Also you need to make sure that you have Ruby version 1.8.6-25 in order to install qtruby. Different versions of Ruby can be targeted from the same system without any problem.
Running Sample Application
QtRuby is bit hard to learn but if you have been doing coding in c++ then you'll prefer QtRuby than any other GUI toolkits. To run a sample code you can use any editor or IDE.
require 'Qt' # Call Qt module app = Qt::Application.new(ARGV) # Instantiate the application button=Qt::PushButton.new("Click this") # Create button with title button.resize(80,30) # resize the button control button.show # Show the button on the app window app.exec # Execute the app (run the mainloop)
In the above sample Qt module is included first. Then window instance of Qt application is created adding a button to it with changed size parameters. Window Qt app is then executed and displayed with above controls.
Pros & Cons
Pros -
- Book for reference available.
- Native looking desktop applications can be created
- Available as a Gem.
Cons -
- Even though a gem is available for the Windows installation, for other platforms, only source code is available.
- Less easy to learn
Projects developed in Qt Ruby
- Google Earth- Google Software to explore virtual world
Shoes
Shoes is a very recently introduced Ruby GUI toolkit. Shoes was designed specifically for Ruby unlike the other toolkits which were first developed for C/C++ developers (like QtRuby). Installing Shoes is very easy and it has packaged set-ups for every platform. Shoes is not really designed for serious,large-scale application but you can write small and useful programs using Shoes. The learning curve for Shoes is very small.
Instead of using widgets like most of the other GUI toolkits, Shoes uses images and text layout. This tiny toolkit is written with the help of an art Engine called Cairo and has very limited native controls.
Installation & getting started
Shoes is probably the simplest and easiest GUI toolkit to install [1]. Once you install Ruby, just download the package from GitHub site and install it on your Windows machine. Once you are done, the interface will give you few options to open the app, package the app or read the manual.
Surprising thing is that we don't even need Ruby or Winzip for running or packaging an app.
Running Sample Application
To run an app in Shoes, you first need to write it using any text editor and then open the app from the integrated app of Shoes. Scope of Shoes is very much limited though.
Shoes.app { @push = button "Click this" # create a button @note = para "Nothing pushed so far" # text to display on the app @push.click { @note.replace "Okay you clicked" # event handler message when button clicked. } }
In the above example, instance of Shoes app is created with a button and a text label. An event handler for button click event is defined. There is no need of run or execute methods which were seen in other sample programs.
Pros & Cons
Pros -
- Good graphics
- Easy control at a lower level
- Simple interface with redistributables and examples
Cons -
- Considered rough around the edges since it attempts to support so many platforms
- Lacks many of the more robust widgets common in other toolkits
Projects developed in Shoes
- Download Organizer - Organize your downloads
- xkcd Scraper Must try app for xkcd lovers
- World Clock Show times for different timezones
Comparison of various GUI toolkits for Ruby
Above are the screen captures of the sample application we made using five GUI toolkits for Ruby. It was found that the QtRuby and Shoes almost imitate the native interface of Windows.
Cross platform comparison
GUI Toolkit | Windows | Linux | Mac OS X |
---|---|---|---|
Tk | yes | yes | yes |
FxRuby | yes | yes | yes |
WxRuby | yes | yes | yes |
QtRuby | yes | yes | yes |
Shoes | yes | yes | yes |
Swing | yes | yes | yes |
Monkeybars | yes | yes | yes |
Cocoa | No | No | yes |
GTK | yes | yes | yes |
Rapid GUI development comparison
While installing the GUI toolkits for Ruby we did a research on the ease of use of above mentioned Toolkit. Some of the toolkit were very easy to install and use while others took so much time asking to install dependencies first. Also, while coding a sample application in these toolkit we compared the level of difficulty of using these toolkit and look and feel of the app which gets developed.
This may be helpful for people to choose the GUI toolkit for Ruby. We assume that Ruby is already installed on the system with ruby gems.
GUI Toolkit | Time taken to build first application | Level of difficulty | Look and feel of interface |
---|---|---|---|
Tk | 18mins | Medium | Average |
FxRuby | 13 mins | Medium | Good |
WxRuby | 11 mins | Medium | Average |
QtRuby | 22 mins | Difficult | Very Good |
Shoes | 8 mins | Easy | Good |
Best GUI Toolkit for Ruby Survey 2008
Towards the end of year 2008, an interesting survey [2] of Ruby programmers was conducted.The set of respondents in the survey were those who had done GUI programming in Ruby, those who had done some Ruby GUI programming in the past but now were not doing so and those who are doing so presently. This survey aimed to find the use and their attitudes towards graphical user interface (GUI) libraries used for creating Ruby desktop applications.Given below are the summarized results as presented by the surveyors, which will help us to get an insight into what were the most important features programmers looked for:
- The most used toolkits were Shoes (21%), Ruby-GNOME2 (19%) and wxRuby (16%).
- In response to a single preferred toolkit, Ruby-GNOME2 and Shoes were chosen by 26%, wxRuby by 17% and RubyCocoa 11%; no other toolkit received more than 10%.
- Ruby-GNOME2 came as the most preferred toolkit by a clear majority (56%)of Japanese Ruby developers, whereas Euro-Americans preferred Shoes and WxRuby followed by Ruby-Gnome.
- Ruby-Tk received the most poor rating for how well it meets users' GUI requirements.
- The majority saw Ruby as a viable GUI programming language, but the unsophistication of the toolkits is the most common reason for not using Ruby for GUI development.
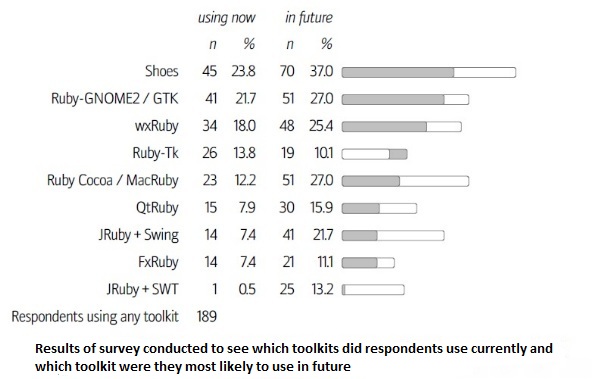
The numbers above clearly show that GUI library usage in Ruby remains very varied. This variation is likely to continue. The situation may turn any side since a lot of new options, such as JRuby + Swing, MacRuby and Shoes are in the competition.
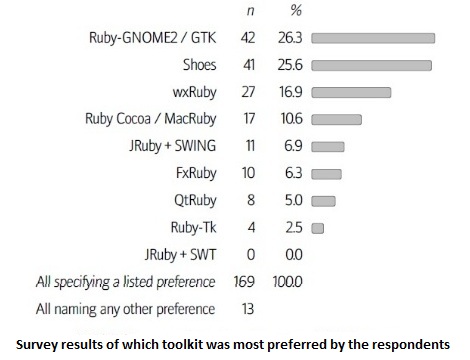
Patterns of preference broadly follow patterns of usage. A very strong relationship between mother tongue and preferred toolkit was observed. Among Japanese Ruby developers, Ruby-GNOME2 was a popular choice and among Europeans, Ruby-Gnome was less popular than Shoes and WxRuby. If the lead developers speak the developer's language, then it is more than likely to present more documentation and active community support in that language. This tells us that even non-technical factors in toolkit selection play an important role.
A guide to 'when to use what'
If you just want to make a message box pop-up occasionally, or ask a user for some simple input, then any of the toolkits will work well.
You may decide to take into consideration characteristics like platform availability, a suitable range of widgets, and appropriate cost if your application requirements are really simple.
For distributing your application, you will need to look into toolkit licensing issues. You will also have to take into consideration whether the user already has the required environment or you will have to create an installation package for all libraries and widgets needed.
For complex applications, which consist of more than a few simple forms, you will definitely require a form-designer tool. You would also need a rich and varied set of available widgets. Instead of re-inventing the wheel, it is better to use or rather re-use an existing component like a date picker or file browser rather than writing your own.
Selection Criteria of GUI toolkit
- Ease of distributing applications
- Web-based/paper based documentation (“how-to-use-this” narrative))
- Availability on multiple platforms.(Great results on Windows, where most of the customers are)
- Maturity / stability (Robust, production-grade,No beta, no pre-1.0)
- Appearance / aesthetics (Looks like 2010 and not 1990)
- Nonrestrictive license (License compatible for commercial use)
- API programming style (Consistent and comprehensible API, with API reference)
- Ease of installation
- Rich set of widgets or components(Features beyond what is readily possible to perform in a web application)
- Community support (Whether or not it is actively maintained and updated)
- Speed / performance
- Internationalization support
- Accessibility features
- Affordable cost
- Existing frameworks and libraries to speed development
- Testing tools and frameworks
Conclusion
Ruby has no real “Ruby-like” GUI system of itself.Tk is the default that comes with Ruby distribution. You can use it if you are fine with just basic functionality and do not care much about appearance. Incase you want something better, you have several choices that we saw. Developing a GUI library for Ruby is actually tedious. There are many reasons for this like, the large number of classes and methods often involved in the implementation; the need to employ lower-level compiled languages;cross-platform support;automated testing; highly variable paths through code; and the complexity of reconciling Ruby's GC-based memory management with that of the base language (often C or C++) in long-running applications.
Just like a coin has two sides, each of them has its share of good qualities, but none of them emerged as a clear winner. There is no unanimous choice for general Ruby cross-platform desktop development. To varying degrees, they all have issues with installation, documentation, design tools, packaging, and deployment.
References
[1] Ruby language
[2] Fowler, C., The Ruby FAQ, www.rubygarden.org/faq
[4] Vinoski, S.; , "Ruby Extensions," Internet Computing, IEEE , vol.10, no.5, pp.85-87, Sept.-Oct. 2006
[5] Cross platform GUI toolkits
[6] 2008 GUI survey
[7] Dave Thomas , Chad Fowler , Andy Hunt, Programming Ruby 1.9: The Pragmatic Programmers' Guide, Pragmatic Bookshelf, 2009
[8] Rapid GUI Development with QtRuby
[9] Wikipedia, Ruby programming language, en.wikipedia.org/wiki/Ruby_programming_language
[11] Ruby TK Guide
[13] FxRuby sample applications
[14] QtRuby Hello world Application
[15] Ruby Shoes toolkit sample